Python Projects: Read public data returned from URL, and parsing JSON to dictionary object
Python Project-10 with Solution
Create a Python project to read public data returned from URL, and parsing JSON to dictionary object.
Sample Solution:
Python Code:
main.py
#Source: https://bit.ly/3eJPW8E
import WebData
data = WebData.WebData("https://data.townofcary.org/api/v2/catalog/datasets/rdu-weather-history")
print("WebData Type:")
print(type(data.parseJson()))
print("\nWebData:")
print(data.parseJson())
Flowchart:
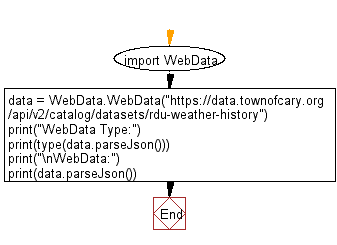
WebData.py
from urllib.request import urlopen
import json
class WebData():
"""
WebData class for reading data returned from URL, and parsing JSON to dictionary object.
"""
def __init__(self, url):
"""
Constructor method.
URL is a string URL, to read data from it.
"""
self._url = url
self._data = None
self._readData()
def _readData(self):
"""
reading data from a URL.
Raises:
ConnectionError: If received an error from server and data couldn't be fetched.
"""
webUrl = urlopen(self._url)
if (webUrl.getcode() == 200):
self._data = webUrl.read().decode('utf-8')
else:
raise ConnectionError("Received an error {} from server, cannot retrieve results ".format(str(webUrl.getcode())))
return self
def getData(self):
"""
Returns the resulting data as a string.
"""
return self._data
def parseJson(self):
"""
Parses the string JSON data to a dictionary object, and returns it.
"""
return json.loads(self.getData())
Output:
WebData Type: <class 'dict'> WebData: {'links': [{'href': 'https://data.townofcary.org/api/v2/catalog/datasets/rdu-weather-history', 'rel': 'self'}, {'href': 'https://data.townofcary.org/api/v2/catalog/datasets', 'rel': 'datasets'}, {'href': 'https://data.townofcary.org/api/v2/catalog/datasets/rdu-weather-history/records', 'rel': 'records'}, {'href': 'https://data.townofcary.org/api/v2/catalog/datasets/rdu-weather-history/exports', 'rel': 'exports'}, {'href': 'https://data.townofcary.org/api/v2/catalog/datasets/rdu-weather-history/aggregates', 'rel': 'aggregate'}, {'href': 'https://data.townofcary.org/api/v2/catalog/datasets/rdu-weather-history/facets', 'rel': 'facets'}, {'href': 'https://data.townofcary.org/api/v2/catalog/datasets/rdu-weather-history/reuses', 'rel': 'reuses'}], 'dataset': {'dataset_id': 'rdu-weather-history', 'dataset_uid': 'da_i2m3v1', 'attachments': [], 'has_records': True, 'data_visible': True, 'fields': [{'description': {'ru': 'Date of Observations', 'fr': 'Date of Observations', 'en': 'Date of Observations', 'nl': 'Date of Observations', 'pt': 'Date of Observations', 'no': 'Date of Observations', 'ca': 'Date of Observations', 'de': 'Date of Observations', 'sv': 'Date of Observations', 'it': 'Date of Observations', 'fa': 'Date of Observations', 'ar': 'Date of Observations', 'eu': 'Date of Observations', 'fi': 'Date of Observations', 'es': 'Date of Observations'}, 'label': 'Date', 'type': 'date', 'name': 'date', 'annotations': {'facet': [], 'facetsort': ['-alphanum'], 'id': [], 'timeserie_precision': ['day']}}, {'description': {'ru': 'Low Temperature of the day in Fahrenheit', 'fr': 'Low Temperature of the day in Fahrenheit', 'en': 'Low Temperature of the day in Fahrenheit', 'nl': 'Low Temperature of the day in Fahrenheit', 'pt': 'Low Temperature of the day in Fahrenheit', 'no': 'Low Temperature of the day in Fahrenheit', 'ca': 'Low Temperature of the day in Fahrenheit', 'de': 'Low Temperature of the day in Fahrenheit', 'sv': 'Low Temperature of the day in Fahrenheit', 'it': 'Low Temperature of the day in Fahrenheit', 'fa': 'Low Temperature of the day in Fahrenheit', 'ar': 'Low Temperature of the day in Fahrenheit', 'eu': 'Low Temperature of the day in Fahrenheit', 'fi': 'Low Temperature of the day in Fahrenheit', 'es': 'Low Temperature of the day in Fahrenheit'}, 'label': 'TemperatureMin', 'type': 'text', 'name': 'temperaturemin', 'annotations': {}}, {'description': {'ru': 'High Temperature of the day in Fahrenheit ', 'fr': 'High Temperature of the day in Fahrenheit ', 'en': 'High Temperature of the day in Fahrenheit ', 'nl': 'High Temperature of the day in Fahrenheit ', 'pt': 'High Temperature of the day in Fahrenheit ', 'no': 'High Temperature of the day in Fahrenheit ', 'ca': 'High Temperature of the day in Fahrenheit ', 'de': 'High Temperature of the day in Fahrenheit ', 'sv': 'High Temperature of the day in Fahrenheit ', 'it': 'High Temperature of the day in Fahrenheit ', 'fa': 'High Temperature of the day in Fahrenheit ', 'ar': 'High Temperature of the day in Fahrenheit ', 'eu': 'High Temperature of the day in Fahrenheit ', 'fi': 'High Temperature of the day in Fahrenheit ', 'es': 'High Temperature of the day in Fahrenheit '}, 'label': 'TemperatureMax', 'type': 'text', 'name': 'temperaturemax', 'annotations': {}}, {'description': {'ru': 'The amount of precipitation in inches', 'fr': 'The amount of precipitation in inches', 'en': 'The amount of precipitation in inches', 'nl': 'The amount of precipitation in inches', 'pt': 'The amount of precipitation in inches', 'no': 'The amount of precipitation in inches', 'ca': 'The amount of precipitation in inches', 'de': 'The amount of precipitation in inches', 'sv': 'The amount of precipitation in inches', 'it': 'The amount of precipitation in inches', 'fa': 'The amount of precipitation in inches', 'ar': 'The amount of precipitation in inches', 'eu': 'The amount of precipitation in inches', 'fi': 'The amount of precipitation in inches', 'es': 'The amount of precipitation in inches'}, 'label': 'Precipitation', 'type': 'text', 'name': 'precipitation', 'annotations': {}}, {'description': {'ru': 'The amount of snowfall in inches', 'fr': 'The amount of snowfall in inches', 'en': 'The amount of snowfall in inches', 'nl': 'The amount of snowfall in inches', 'pt': 'The amount of snowfall in inches', 'no': 'The amount of snowfall in inches', 'ca': 'The amount of snowfall in inches', 'de': 'The amount of snowfall in inches', 'sv': 'The amount of snowfall in inches', 'it': 'The amount of snowfall in inches', 'fa': 'The amount of snowfall in inches', 'ar': 'The amount of snowfall in inches', 'eu': 'The amount of snowfall in inches', 'fi': 'The amount of snowfall in inches', 'es': 'The amount of snowfall in inches'}, 'label': 'Snowfall', 'type': 'text', 'name': 'snowfall', 'annotations': {}}, {'description': {'ru': 'Depth of Snow in inches', 'fr': 'Depth of Snow in inches', 'en': 'Depth of Snow in inches', 'nl': 'Depth of Snow in inches', 'pt': 'Depth of Snow in inches', 'no': 'Depth of Snow in inches', 'ca': 'Depth of Snow in inches', 'de': 'Depth of Snow in inches', 'sv': 'Depth of Snow in inches', 'it': 'Depth of Snow in inches', 'fa': 'Depth of Snow in inches', 'ar': 'Depth of Snow in inches', 'eu': 'Depth of Snow in inches', 'fi': 'Depth of Snow in inches', 'es': 'Depth of Snow in inches'}, 'label': 'SnowDepth', 'type': 'text', 'name': 'snowdepth', 'annotations': {}}, {'description': {'ru': 'Average Wind Speed (Miles Per Hour)', 'fr': 'Average Wind Speed (Miles Per Hour)', 'en': 'Average Wind Speed (Miles Per Hour)', 'nl': 'Average Wind Speed (Miles Per Hour)', 'pt': 'Average Wind Speed (Miles Per Hour)', 'no': 'Average Wind Speed (Miles Per Hour)', 'ca': 'Average Wind Speed (Miles Per Hour)', 'de': 'Average Wind Speed (Miles Per Hour)', 'sv': 'Average Wind Speed (Miles Per Hour)', 'it': 'Average Wind Speed (Miles Per Hour)', 'fa': 'Average Wind Speed (Miles Per Hour)', 'ar': 'Average Wind Speed (Miles Per Hour)', 'eu': 'Average Wind Speed (Miles Per Hour)', 'fi': 'Average Wind Speed (Miles Per Hour)', 'es': 'Average Wind Speed (Miles Per Hour)'}, 'label': 'AvgWindSpeed', 'type': 'text', 'name': 'avgwindspeed', 'annotations': {}}, {'description': {'ru': 'Direction of fastest 2-minute wind (degrees)', 'fr': 'Direction of fastest 2-minute wind (degrees)', 'en': 'Direction of fastest 2-minute wind (degrees)', 'nl': 'Direction of fastest 2-minute wind (degrees)', 'pt': 'Direction of fastest 2-minute wind (degrees)', 'no': 'Direction of fastest 2-minute wind (degrees)', 'ca': 'Direction of fastest 2-minute wind (degrees)', 'de': 'Direction of fastest 2-minute wind (degrees)', 'sv': 'Direction of fastest 2-minute wind (degrees)', 'it': 'Direction of fastest 2-minute wind (degrees)', 'fa': 'Direction of fastest 2-minute wind (degrees)', 'ar': 'Direction of fastest 2-minute wind (degrees)', 'eu': 'Direction of fastest 2-minute wind (degrees)', 'fi': 'Direction of fastest 2-minute wind (degrees)', 'es': 'Direction of fastest 2-minute wind (degrees)'}, 'label': 'Fastest2MinWindDir', 'type': 'text', 'name': 'fastest2minwinddir', 'annotations': {}}, {'description': {'ru': 'Fastest 2-minute wind speed (MPH)', 'fr': 'Fastest 2-minute wind speed (MPH)', 'en': 'Fastest 2-minute wind speed (MPH)', 'nl': 'Fastest 2-minute wind speed (MPH)', 'pt': 'Fastest 2-minute wind speed (MPH)', 'no': 'Fastest 2-minute wind speed (MPH)', 'ca': 'Fastest 2-minute wind speed (MPH)', 'de': 'Fastest 2-minute wind speed (MPH)', 'sv': 'Fastest 2-minute wind speed (MPH)', 'it': 'Fastest 2-minute wind speed (MPH)', 'fa': 'Fastest 2-minute wind speed (MPH)', 'ar': 'Fastest 2-minute wind speed (MPH)', 'eu': 'Fastest 2-minute wind speed (MPH)', 'fi': 'Fastest 2-minute wind speed (MPH)', 'es': 'Fastest 2-minute wind speed (MPH)'}, 'label': 'Fastest2MinWindSpeed', 'type': 'text', 'name': 'fastest2minwindspeed', 'annotations': {}}, {'description': {'ru': 'Direction of fastest 5 second wind (degrees)', 'fr': 'Direction of fastest 5 second wind (degrees)', 'en': 'Direction of fastest 5 second wind (degrees)', 'nl': 'Direction of fastest 5 second wind (degrees)', 'pt': 'Direction of fastest 5 second wind (degrees)', 'no': 'Direction of fastest 5 second wind (degrees)', 'ca': 'Direction of fastest 5 second wind (degrees)', 'de': 'Direction of fastest 5 second wind (degrees)', 'sv': 'Direction of fastest 5 second wind (degrees)', 'it': 'Direction of fastest 5 second wind (degrees)', 'fa': 'Direction of fastest 5 second wind (degrees)', 'ar': 'Direction of fastest 5 second wind (degrees)', 'eu': 'Direction of fastest 5 second wind (degrees)', 'fi': 'Direction of fastest 5 second wind (degrees)', 'es': 'Direction of fastest 5 second wind (degrees)'}, 'label': 'Fastest5SecWindDir', 'type': 'text', 'name': 'fastest5secwinddir', 'annotations': {}}, {'description': {'ru': 'Fastest 5-second wind speed (MPH)', 'fr': 'Fastest 5-second wind speed (MPH)', 'en': 'Fastest 5-second wind speed (MPH)', 'nl': 'Fastest 5-second wind speed (MPH)', 'pt': 'Fastest 5-second wind speed (MPH)', 'no': 'Fastest 5-second wind speed (MPH)', 'ca': 'Fastest 5-second wind speed (MPH)', 'de': 'Fastest 5-second wind speed (MPH)', 'sv': 'Fastest 5-second wind speed (MPH)', 'it': 'Fastest 5-second wind speed (MPH)', 'fa': 'Fastest 5-second wind speed (MPH)', 'ar': 'Fastest 5-second wind speed (MPH)', 'eu': 'Fastest 5-second wind speed (MPH)', 'fi': 'Fastest 5-second wind speed (MPH)', 'es': 'Fastest 5-second wind speed (MPH)'}, 'label': 'Fastest5SecWindSpeed', 'type': 'text', 'name': 'fastest5secwindspeed', 'annotations': {}}, {'description': {'ru': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'fr': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'en': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'nl': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'pt': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'no': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'ca': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'de': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'sv': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'it': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'fa': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'ar': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'eu': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'fi': 'Fog, ice fog, or freezing fog (may include heavy fog)', 'es': 'Fog, ice fog, or freezing fog (may include heavy fog)'}, 'label': 'Fog', 'type': 'text', 'name': 'fog', 'annotations': {}}, {'description': {'ru': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'fr': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'en': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'nl': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'pt': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'no': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'ca': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'de': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'sv': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'it': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'fa': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'ar': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'eu': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'fi': 'Heavy fog or heaving freezing fog (not always distinguished from fog)', 'es': 'Heavy fog or heaving freezing fog (not always distinguished from fog)'}, 'label': 'FogHeavy', 'type': 'text', 'name': 'fogheavy', 'annotations': {}}, {'description': {'ru': 'Mist?', 'fr': 'Mist?', 'en': 'Mist?', 'nl': 'Mist?', 'pt': 'Mist?', 'no': 'Mist?', 'ca': 'Mist?', 'de': 'Mist?', 'sv': 'Mist?', 'it': 'Mist?', 'fa': 'Mist?', 'ar': 'Mist?', 'eu': 'Mist?', 'fi': 'Mist?', 'es': 'Mist?'}, 'label': 'Mist', 'type': 'text', 'name': 'mist', 'annotations': {}}, {'description': {'ru': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'fr': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'en': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'nl': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'pt': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'no': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'ca': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'de': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'sv': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'it': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'fa': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'ar': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'eu': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'fi': 'Rain (may include freezing rain, drizzle, and freezing drizzle)', 'es': 'Rain (may include freezing rain, drizzle, and freezing drizzle)'}, 'label': 'Rain', 'type': 'text', 'name': 'rain', 'annotations': {}}, {'description': {'ru': 'Ground Fog', 'fr': 'Ground Fog', 'en': 'Ground Fog', 'nl': 'Ground Fog', 'pt': 'Ground Fog', 'no': 'Ground Fog', 'ca': 'Ground Fog', 'de': 'Ground Fog', 'sv': 'Ground Fog', 'it': 'Ground Fog', 'fa': 'Ground Fog', 'ar': 'Ground Fog', 'eu': 'Ground Fog', 'fi': 'Ground Fog', 'es': 'Ground Fog'}, 'label': 'FogGround', 'type': 'text', 'name': 'fogground', 'annotations': {}}, {'description': {'ru': 'Ice pellets, sleet, snow pellets, or small hail', 'fr': 'Ice pellets, sleet, snow pellets, or small hail', 'en': 'Ice pellets, sleet, snow pellets, or small hail', 'nl': 'Ice pellets, sleet, snow pellets, or small hail', 'pt': 'Ice pellets, sleet, snow pellets, or small hail', 'no': 'Ice pellets, sleet, snow pellets, or small hail', 'ca': 'Ice pellets, sleet, snow pellets, or small hail', 'de': 'Ice pellets, sleet, snow pellets, or small hail', 'sv': 'Ice pellets, sleet, snow pellets, or small hail', 'it': 'Ice pellets, sleet, snow pellets, or small hail', 'fa': 'Ice pellets, sleet, snow pellets, or small hail', 'ar': 'Ice pellets, sleet, snow pellets, or small hail', 'eu': 'Ice pellets, sleet, snow pellets, or small hail', 'fi': 'Ice pellets, sleet, snow pellets, or small hail', 'es': 'Ice pellets, sleet, snow pellets, or small hail'}, 'label': 'Ice', 'type': 'text', 'name': 'ice', 'annotations': {}}, {'description': {'ru': 'Glaze or rime', 'fr': 'Glaze or rime', 'en': 'Glaze or rime', 'nl': 'Glaze or rime', 'pt': 'Glaze or rime', 'no': 'Glaze or rime', 'ca': 'Glaze or rime', 'de': 'Glaze or rime', 'sv': 'Glaze or rime', 'it': 'Glaze or rime', 'fa': 'Glaze or rime', 'ar': 'Glaze or rime', 'eu': 'Glaze or rime', 'fi': 'Glaze or rime', 'es': 'Glaze or rime'}, 'label': 'Glaze', 'type': 'text', 'name': 'glaze', 'annotations': {}}, {'description': {'ru': 'Drizzle', 'fr': 'Drizzle', 'en': 'Drizzle', 'nl': 'Drizzle', 'pt': 'Drizzle', 'no': 'Drizzle', 'ca': 'Drizzle', 'de': 'Drizzle', 'sv': 'Drizzle', 'it': 'Drizzle', 'fa': 'Drizzle', 'ar': 'Drizzle', 'eu': 'Drizzle', 'fi': 'Drizzle', 'es': 'Drizzle'}, 'label': 'Drizzle', 'type': 'text', 'name': 'drizzle', 'annotations': {}}, {'description': {'ru': 'Snow, snow pellets, snow grains, or ice crystals', 'fr': 'Snow, snow pellets, snow grains, or ice crystals', 'en': 'Snow, snow pellets, snow grains, or ice crystals', 'nl': 'Snow, snow pellets, snow grains, or ice crystals', 'pt': 'Snow, snow pellets, snow grains, or ice crystals', 'no': 'Snow, snow pellets, snow grains, or ice crystals', 'ca': 'Snow, snow pellets, snow grains, or ice crystals', 'de': 'Snow, snow pellets, snow grains, or ice crystals', 'sv': 'Snow, snow pellets, snow grains, or ice crystals', 'it': 'Snow, snow pellets, snow grains, or ice crystals', 'fa': 'Snow, snow pellets, snow grains, or ice crystals', 'ar': 'Snow, snow pellets, snow grains, or ice crystals', 'eu': 'Snow, snow pellets, snow grains, or ice crystals', 'fi': 'Snow, snow pellets, snow grains, or ice crystals', 'es': 'Snow, snow pellets, snow grains, or ice crystals'}, 'label': 'Snow', 'type': 'text', 'name': 'snow', 'annotations': {}}, {'description': {'ru': 'Freezing rain', 'fr': 'Freezing rain', 'en': 'Freezing rain', 'nl': 'Freezing rain', 'pt': 'Freezing rain', 'no': 'Freezing rain', 'ca': 'Freezing rain', 'de': 'Freezing rain', 'sv': 'Freezing rain', 'it': 'Freezing rain', 'fa': 'Freezing rain', 'ar': 'Freezing rain', 'eu': 'Freezing rain', 'fi': 'Freezing rain', 'es': 'Freezing rain'}, 'label': 'FreezingRain', 'type': 'text', 'name': 'freezingrain', 'annotations': {}}, {'description': {'ru': 'Smoke or haze', 'fr': 'Smoke or haze', 'en': 'Smoke or haze', 'nl': 'Smoke or haze', 'pt': 'Smoke or haze', 'no': 'Smoke or haze', 'ca': 'Smoke or haze', 'de': 'Smoke or haze', 'sv': 'Smoke or haze', 'it': 'Smoke or haze', 'fa': 'Smoke or haze', 'ar': 'Smoke or haze', 'eu': 'Smoke or haze', 'fi': 'Smoke or haze', 'es': 'Smoke or haze'}, 'label': 'SmokeHaze', 'type': 'text', 'name': 'smokehaze', 'annotations': {}}, {'description': {'ru': 'Thunder', 'fr': 'Thunder', 'en': 'Thunder', 'nl': 'Thunder', 'pt': 'Thunder', 'no': 'Thunder', 'ca': 'Thunder', 'de': 'Thunder', 'sv': 'Thunder', 'it': 'Thunder', 'fa': 'Thunder', 'ar': 'Thunder', 'eu': 'Thunder', 'fi': 'Thunder', 'es': 'Thunder'}, 'label': 'Thunder', 'type': 'text', 'name': 'thunder', 'annotations': {}}, {'description': {'ru': 'High or damaging winds', 'fr': 'High or damaging winds', 'en': 'High or damaging winds', 'nl': 'High or damaging winds', 'pt': 'High or damaging winds', 'no': 'High or damaging winds', 'ca': 'High or damaging winds', 'de': 'High or damaging winds', 'sv': 'High or damaging winds', 'it': 'High or damaging winds', 'fa': 'High or damaging winds', 'ar': 'High or damaging winds', 'eu': 'High or damaging winds', 'fi': 'High or damaging winds', 'es': 'High or damaging winds'}, 'label': 'HighWind', 'type': 'text', 'name': 'highwind', 'annotations': {}}, {'description': {'ru': 'Hail (may include small hail)', 'fr': 'Hail (may include small hail)', 'en': 'Hail (may include small hail)', 'nl': 'Hail (may include small hail)', 'pt': 'Hail (may include small hail)', 'no': 'Hail (may include small hail)', 'ca': 'Hail (may include small hail)', 'de': 'Hail (may include small hail)', 'sv': 'Hail (may include small hail)', 'it': 'Hail (may include small hail)', 'fa': 'Hail (may include small hail)', 'ar': 'Hail (may include small hail)', 'eu': 'Hail (may include small hail)', 'fi': 'Hail (may include small hail)', 'es': 'Hail (may include small hail)'}, 'label': 'Hail', 'type': 'text', 'name': 'hail', 'annotations': {}}, {'description': {'ru': 'Blowing or drifting snow', 'fr': 'Blowing or drifting snow', 'en': 'Blowing or drifting snow', 'nl': 'Blowing or drifting snow', 'pt': 'Blowing or drifting snow', 'no': 'Blowing or drifting snow', 'ca': 'Blowing or drifting snow', 'de': 'Blowing or drifting snow', 'sv': 'Blowing or drifting snow', 'it': 'Blowing or drifting snow', 'fa': 'Blowing or drifting snow', 'ar': 'Blowing or drifting snow', 'eu': 'Blowing or drifting snow', 'fi': 'Blowing or drifting snow', 'es': 'Blowing or drifting snow'}, 'label': 'BlowingSnow', 'type': 'text', 'name': 'blowingsnow', 'annotations': {}}, {'description': {'ru': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'fr': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'en': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'nl': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'pt': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'no': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'ca': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'de': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'sv': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'it': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'fa': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'ar': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'eu': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'fi': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction', 'es': 'Dust, volcanic ash, blowing dust, blowing sand, or blowing obstruction'}, 'label': 'Dust', 'type': 'text', 'name': 'dust', 'annotations': {}}, {'description': {'ru': 'Ice fog or freezing fog', 'fr': 'Ice fog or freezing fog', 'en': 'Ice fog or freezing fog', 'nl': 'Ice fog or freezing fog', 'pt': 'Ice fog or freezing fog', 'no': 'Ice fog or freezing fog', 'ca': 'Ice fog or freezing fog', 'de': 'Ice fog or freezing fog', 'sv': 'Ice fog or freezing fog', 'it': 'Ice fog or freezing fog', 'fa': 'Ice fog or freezing fog', 'ar': 'Ice fog or freezing fog', 'eu': 'Ice fog or freezing fog', 'fi': 'Ice fog or freezing fog', 'es': 'Ice fog or freezing fog'}, 'label': 'FreezingFog', 'type': 'text', 'name': 'freezingfog', 'annotations': {}}], 'metas': {'default': {'records_count': 0, 'modified': '2016-02-14T02:54:45+00:00', 'source_domain_address': None, 'references': None, 'keyword': ['Weather', 'Rainfall', 'Temperature'], 'source_domain_title': None, 'geographic_reference': None, 'timezone': None, 'title': 'Local Weather Archive', 'parent_domain': None, 'theme': ['Environment'], 'modified_updates_on_data_change': None, 'metadata_processed': '2020-07-20T08:00:11.986000+00:00', 'data_processed': '2020-07-20T06:01:31+00:00', 'territory': None, 'description': '<p>Pull weather data as collected at Raleigh-Durham International Airport by NOAA.</p><p>This dataset contains Raleigh Durham International Airport\xa0weather data pulled from the NOAA\xa0web service described at:</p><p><a href="http://www.ncdc.noaa.gov/cdo-web/webservices/v2">http://www.ncdc.noaa.gov/cdo-web/webservices/v2</a></p><p>We have pulled this data and converted the data to commonly used units.\xa0</p>', 'modified_updates_on_metadata_change': None, 'shared_catalog': None, 'source_domain': None, 'attributions': ['NOAA - NCEI'], 'geographic_area_mode': None, 'geographic_reference_auto': None, 'geographic_area': None, 'publisher': 'National Oceanic and Atmospheric Administration - National Centers for Environmental Information', 'language': 'en', 'license': 'Open Database License (ODbL)', 'source_dataset': None, 'metadata_languages': ['en'], 'oauth_scope': None, 'federated': False, 'license_url': 'http://opendatacommons.org/licenses/odbl/'}}, 'features': ['analyze', 'timeserie']}}
Flowchart:
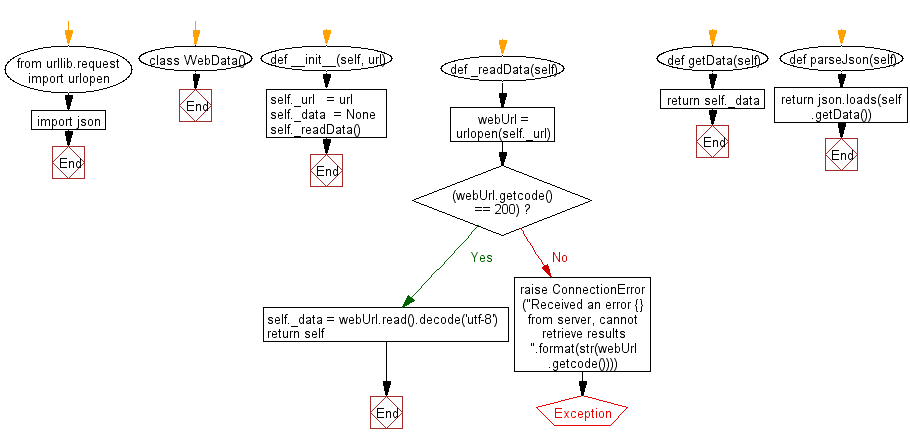
Contribute your code and comments through Disqus.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics