Python Projects: Split a given data list into several small sections
Python Project-8 with Solution
Create a Python project to split a given data list into several small sections.
Sample Solution:
Python Code:
main.py
#Source: https://bit.ly/3hn17pL
from slicer import Slicer
#My list data
data = [
200, 1230, 15, 2200, 5550
]
#2 is amount to split
data_slice = Slicer.cut(data, 2)
print(data_slice)
data = [
{
"foodname": "Ayam Bakar",
"price": 12000
},
{
"foodname": "Jus Mangga",
"price": 7000
},
{
"foodname": "Mie Goreng",
"price": 9000
},
{
"foodname": "Chicken Katsu",
"price": 15000
},
]
print(Slicer.cut(data, 2))
Flowchart:
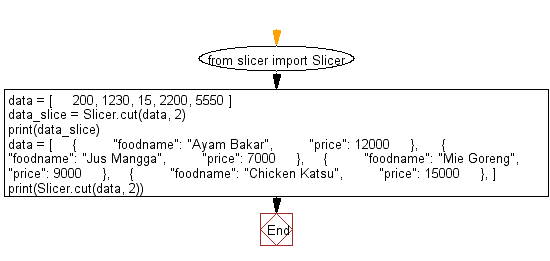
slicer.py
class Slicer(object):
@staticmethod
def cut(list_data, amount):
slices_list, temp_slices = [], []
for x in range(1, len(list_data)+1):
temp_slices.append(list_data[x-1])
if x % amount == 0 or x == len(list_data):
slices_list.append(temp_slices)
temp_slices = []
return slices_list
Output:
[[200, 1230], [15, 2200], [5550]] [[{'foodname': 'Ayam Bakar', 'price': 12000}, {'foodname': 'Jus Mangga', 'price': 7000}], [{'foodname': 'Mie Goreng', 'price': 9000}, {'foodname': 'Chicken Katsu', 'price': 15000}]]
Flowchart:
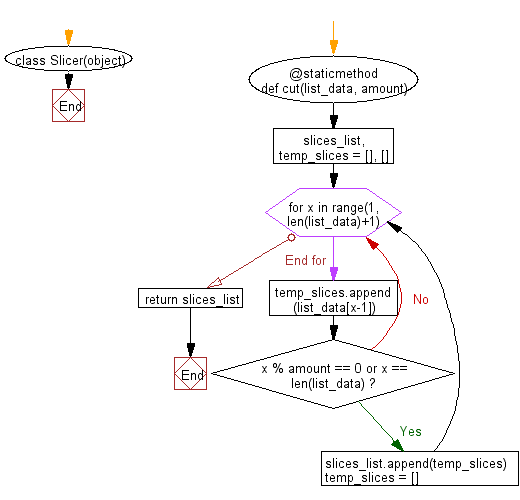
Contribute your code and comments through Disqus.