Printing numbers with a delay using asyncio coroutines in Python
3. Event Loop Number Printer
Write a Python program that creates an asyncio event loop and runs a coroutine that prints numbers from 1 to 7 with a delay of 1 second each.
Sample Solution:
Code:
import asyncio
async def display_numbers():
for i in range(1, 8):
print(i)
await asyncio.sleep(1)
# Run the coroutine using asyncio.run()
asyncio.run(display_numbers())
Output:
1 2 3 4 5 6 7
Explanation:
In the above exercise-
The "display_numbers()" function is an asynchronous coroutine. It uses the 'await' keyword to introduce a pause using await asyncio.sleep(1) after printing each number. This 'sleep()' function introduces a 1 second delay.
The asyncio.run() function runs the “display_numbers()” coroutine. This function is introduced in Python 3.7 and provides a simple and clean way to run an asynchronous function in an event loop.
- Inside the event loop created by asyncio.run(), the display_numbers() coroutine is executed.
- For each iteration of the loop (from 1 to 7), the current number is displayed, and then the coroutine is paused for 1 second using await asyncio.sleep(1).
Flowchart:
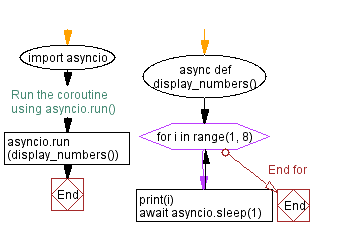
For more Practice: Solve these Related Problems:
- Write a Python program that creates an asyncio event loop to run a coroutine that prints numbers from 1 to 7, with a 1-second delay between each number.
- Write a Python script to implement a coroutine that iterates from 1 to 7, printing each number after a delay, and then use asyncio.run() to execute the coroutine.
- Write a Python function that uses an event loop to schedule a coroutine printing a sequence of numbers from 1 to 7 with fixed delays, and then prints "Done" at the end.
- Write a Python program to create a coroutine that prints numbers 1 to 7 with a 1-second interval and then stops the event loop after completion, printing the total elapsed time.
Go to:
Previous: Running asynchronous Python functions with different time delays.
Next: Fetching data asynchronously from multiple URLs using Python async IO.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.