Python: Sum of all items of a given array of integers where each integer is multiplied by its index
Sum of Items Weighted by Index
Write a Python program to compute the sum of all items in a given array of integers where each integer is multiplied by its index. Return 0 if there is no number.
Sample Solution:
Python Code:
# Define a function named sum_index_multiplier that takes a list of numbers (nums) as an argument.
def sum_index_multiplier(nums):
# Use a generator expression within the sum function to calculate the sum of each element multiplied by its index.
# The expression j*i for i, j in enumerate(nums) iterates over the elements and their indices.
return sum(j * i for i, j in enumerate(nums))
# Test the function with different lists of numbers and print the results.
# Test case 1
print(sum_index_multiplier([1,2,3,4]))
# Test case 2
print(sum_index_multiplier([-1,-2,-3,-4]))
# Test case 3
print(sum_index_multiplier([]))
Sample Output:
20 -20 0
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "sum_index_multiplier()" that takes a list of numbers (nums) as an argument.
- Generator Expression and Enumerate:
- The function uses a generator expression within the "sum()" function to calculate the sum of each element multiplied by its index. The expression j * i for i, j in enumerate(nums) iterates over the elements and their indices.
- Return Statement:
- The function returns the result of the sum operation.
Visual Presentation:
Flowchart:
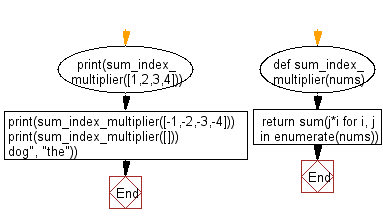
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the position of the second occurrence of a given string in another given string.
Next: Write a Python program to find the name of the oldest student from a given dictionary containing the names and ages of a group of students.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics