Python: Find the name of the oldest student from a given dictionary
Oldest Student Finder
Write a Python program to find the name of the oldest student in a given dictionary containing the names and ages of a group of students.
Sample Solution:
Python Code:
# Define a function named oldest_student that takes a dictionary of students and their ages (students) as an argument.
def oldest_student(students):
# Use the max function with the 'key' argument to find the student with the maximum age.
# The 'key=students.get' argument specifies that the maximum should be determined based on the values (ages) in the dictionary.
return max(students, key=students.get)
# Test the function with different dictionaries of students and ages and print the results.
# Test case 1
print(oldest_student({"Bernita Ahner": 12, "Kristie Marsico": 11,
"Sara Pardee": 14, "Fallon Fabiano": 11,
"Nidia Dominique": 15}))
# Test case 2
print(oldest_student({"Nilda Woodside": 12, "Jackelyn Pineda": 12.2,
"Sofia Park": 12.4, "Joannie Archibald": 12.6,
"Becki Saunder": 12.7}))
Sample Output:
Nidia Dominique Becki Saunder
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "oldest_student()" that takes a dictionary of students and their ages (students) as an argument.
- Max Function with Key:
- The function uses the "max()" function with the 'key' argument to find the student with the maximum age. The key=students.get argument specifies that the maximum should be determined based on the values (ages) in the dictionary.
- Return Statement:
- The function returns the result of the 'max' operation.
Flowchart:
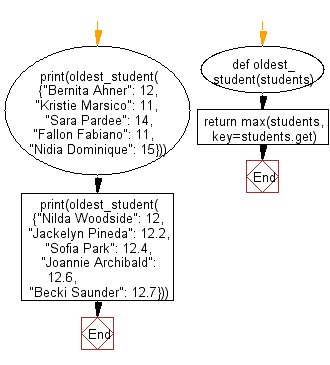
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to compute the sum of all items of a given array of integers where each integer is multiplied by its index.
Next: Write a Python program to create a new string with no duplicate consecutive letters from a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics