Python: Check whether two given lines are parallel or not
Python Basic - 1: Exercise-103 with Solution
Write a Python program to check whether two given lines are parallel or not.
Note: Parallel lines are two or more lines that never intersect. Parallel Lines are like railroad tracks that never intersect.
The General Form of the equation of a straight line is: ax + by = c
The said straight line is represented in a list as [a, b, c]
Example of two parallel lines:
x + 4y = 10 and x + 4y = 14
Sample Solution:
Python Code:
# Define a function named parallel_lines that takes two lines represented by lists (line1 and line2) as arguments.
def parallel_lines(line1, line2):
# Check if the slopes of the two lines are equal.
# The condition line1[0]/line1[1] == line2[0]/line2[1] compares the slopes of the lines.
return line1[0]/line1[1] == line2[0]/line2[1]
# Test the function with different lines represented by lists and print the results.
# Test case 1
# 2x + 3y = 4, 2x + 3y = 8
print(parallel_lines([2,3,4], [2,3,8]))
# Test case 2
# 2x + 3y = 4, 4x - 3y = 8
print(parallel_lines([2,3,4], [4,-3,8]))
Sample Output:
True False
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "parallel_lines()" that takes two lines represented by lists (line1 and line2) as arguments.
- Return Statement:
- The function returns 'True' if the slopes of the two lines are equal, indicating that the lines are parallel.
- Slope comparison:
- The condition line1[0]/line1[1] == line2[0]/line2[1] compares the slopes of the two lines.
- Test cases:
- The function is tested with different lines represented by lists using print(parallel_lines(...)).
Flowchart:
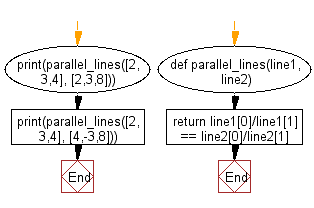
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to create a new string with no duplicate consecutive letters from a given string.
Next: Write a Python program to find a number in a given matrix, which is maximum in its column and minimum in its row.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/basic/python-basic-1-exercise-103.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics