Python: Check whether two given circles are intersecting
Circle Intersection Checker
Write a Python program which checks whether two circles in the same plane (with the same center (x,y) and radius) intersect. If intersection occurs, return true, otherwise return false.
Sample Solution:
Python Code:
# Define a function named is_circle_collision that checks if two circles collide.
def is_circle_collision(circle1, circle2):
# Extract coordinates (x, y) and radius (r) for both circles from the input lists.
x1, y1, r1 = circle1
x2, y2, r2 = circle2
# Calculate the distance between the centers of the two circles using the distance formula.
distance = ((x1 - x2) ** 2 + (y1 - y2) ** 2) ** 0.5
# Check if the distance is less than or equal to the sum of the radii, indicating a collision.
return distance <= r1 + r2
# Test the function with different circles and print the results.
# Test case 1
print(is_circle_collision([1, 2, 4], [1, 2, 8]))
# Test case 2
print(is_circle_collision([0, 0, 2], [10, 10, 5]))
Sample Output:
True False
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "is_circle_collision()" that checks if two circles (represented as lists with coordinates [x, y] and radius [r]) collide.
- Circle Parameters Extraction:
- Extracts the coordinates (x, y) and radius (r) for both circles from the input lists.
- Distance calculation:
- Calculates the distance between the centers of the two circles using the distance formula.
- Collision Check:
- Checks if the distance is less than or equal to the sum of the radii, which indicates a collision.
- Test cases:
- The function is tested with different circles, and the results are printed using print(is_circle_collision(...)).
Flowchart:
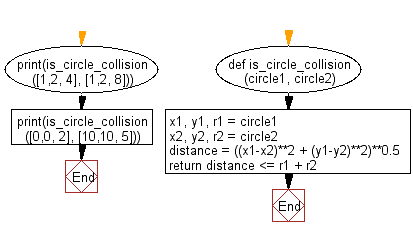
For more Practice: Solve these Related Problems:
- Write a Python program to determine if two circles intersect by comparing the distance between centers with the sum of their radii.
- Write a Python program to check for circle intersection using the Euclidean distance formula on their center coordinates.
- Write a Python program to verify whether two circles overlap, touch, or are separate by computing center distance and radius sum.
- Write a Python program to compute the intersection condition of two circles by comparing their center-to-center distance with their radii.
Go to:
Previous: Write a Python program to remove the duplicate numbers from a given list of numbers.
Next: Write a Python program to compute the digit distance between two integers.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.