Python: Reverse all the words which have even length
Reverse Odd-Length Words
Write a Python program to reverse all words of odd lengths.
Sample Solution:
Python Code:
# Define a function named reverse_even that reverses the even-length words in a given text.
def reverse_even(txt):
# Use a list comprehension to iterate over words in the text.
# Reverse even-length words while leaving odd-length words unchanged.
reversed_words = [i[::-1] if not len(i) % 2 else i for i in txt.split()]
# Join the modified words into a single string separated by spaces.
return ' '.join(reversed_words)
# Test the function with different text inputs and print the results.
# Test case 1
print(reverse_even("The quick brown fox jumps over the lazy dog"))
# Test case 2
print(reverse_even("Python Exercises"))
Sample Output:
The quick brown fox jumps revo the yzal dog nohtyP Exercises
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "reverse_even()" that reverses the even-length words in a given text.
- Word reversal:
- A list comprehension is used to iterate over words in the text.
- For each word, it checks if the length is even (len(i) % 2 == 0). If so, it reverses the word; otherwise, it leaves it unchanged.
- Joining Modified Words:
- The modified words are joined into a single string using ' '.join(...).
Visual Presentation:
Flowchart:
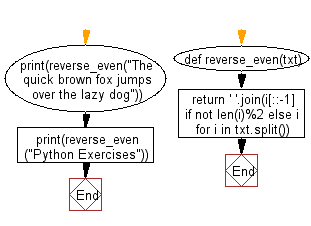
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to compute the digit distance between two integers.
Next: Write a Python program to print letters from the English alphabet from a-z and A-Z.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics