Python: Create a coded string from a given string, using specified formula
String Encoding with Rules
Write a Python program to create a coded string from a given string, using a specified formula.
Replace all 'P' with '9', 'T' with '0', 'S' with '1', 'H' with '6' and 'A' with '8'
Sample Solution-1:
Python Code:
# Define a function 'test' that translates characters in the input string based on a mapping.
def test(input_str):
# Use the 'translate' method along with 'str.maketrans' to create a mapping and perform translation.
translated_str = input_str.translate(str.maketrans('PTSHA', '90168'))
return translated_str
# Test the 'test' function with different input strings.
str1 = "PHP"
print("Original string: ", str1)
print("Coded string: ", test(str1))
str2 = "JAVASCRIPT"
print("\nOriginal string: ", str2)
print("Coded string: ", test(str2))
Sample Output:
Original string: PHP Coded string: 969 Original string: JAVASCRIPT Coded string: J8V81CRI90
Explanation:
Here is a breakdown of the above Python code:
- Translation function (test function):
- The "test()" function is defined to perform character translation in an input string.
- It uses the str.translate method along with str.maketrans to create a mapping and perform the translation.
Flowchart:
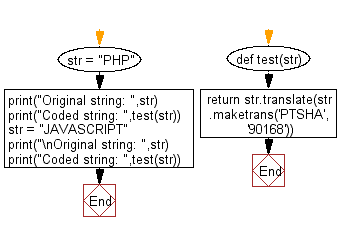
Sample Solution-2:
Python Code:
# Define a function 'test' that replaces specific characters in the input string with predefined values.
def test(input_str):
# Use the 'replace' method to replace each specified character with its corresponding value.
translated_str = input_str.replace('P', '9').replace('T', '0').replace('S', '1').replace('H', '6').replace('A', '8')
return translated_str
# Test the 'test' function with different input strings.
str1 = "PHP"
print("Original string: ", str1)
print("Coded string: ", test(str1))
str2 = "JAVASCRIPT"
print("\nOriginal string: ", str2)
print("Coded string: ", test(str2))
Sample Output:
Original string: PHP Coded string: 969 Original string: JAVASCRIPT Coded string: J8V81CRI90
Explanation:
Here is a breakdown of the above Python code:
- Translation Function (test function):
- The "test()" function is defined to replace specific characters in an input string with predefined values.
- It uses the "replace" method to replace each occurrence of the specified characters with their corresponding values.
Flowchart:
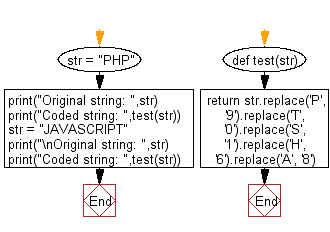
For more Practice: Solve these Related Problems:
- Write a Python program to encode a string by replacing specified characters with designated numbers according to given rules.
- Write a Python program to transform a string using a mapping dictionary that substitutes certain letters with digits.
- Write a Python program to perform character substitution in a string based on predefined encoding rules.
- Write a Python program to create a coded string by iterating over each character and applying specific replacement rules.
Go to:
Previous: Write a Python program to calculate Euclid's totient function of a given integer. Use a primitive method to calculate Euclid's totient function.
Next: Write a Python program to check if a given string contains only lowercase or uppercase characters.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.