Python: Check if a given string contains two similar consecutive letters
Python Basic - 1: Exercise-124 with Solution
Write a Python program to check if a given string contains two similar consecutive letters.
Sample Solution-1:
Python Code:
# Define a function 'test' that checks if there are consecutive similar letters in a string.
def test(input_str):
# Use the 'any' function along with a generator expression to check for consecutive similar letters using 'zip'.
return any(c1 == c2 for c1, c2 in zip(input_str, input_str[1:]))
# Test the 'test' function with different input strings.
str1 = "PHP"
print("Original string: ", str1)
print("Check for consecutive similar letters! ", test(str1))
str2 = "PHHP"
print("\nOriginal string: ", str2)
print("Check for consecutive similar letters! ", test(str2))
str3 = "PHPP"
print("\nOriginal string: ", str3)
print("Check for consecutive similar letters! ", test(str3))
Sample Output:
Original string: PHP Check for consecutive similar letters! False Original string: PHHP Check for consecutive similar letters! True Original string: PHPP Check for consecutive similar letters! True
Explanation:
Here is a breakdown of the above Python code:
- Test Function (test function):
- The "test()" function is defined to check if there are consecutive similar letters in a given string.
- It uses the "any()" function with a generator expression inside 'zip' to compare each character with its consecutive character in the string.
Flowchart:
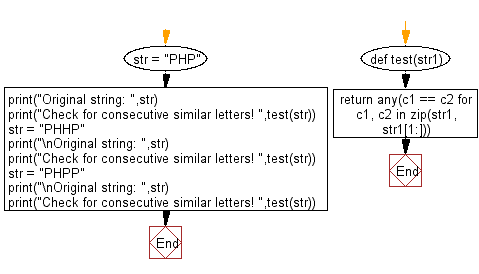
Sample Solution-2:
Python Code:
# Define a function 'test' that checks if there are consecutive similar letters in a string.
def test(input_str):
# Iterate through each character in the input string.
for el in input_str:
# Check if the current character repeated twice is present in the input string.
if el * 2 in input_str:
return True
# If no consecutive similar letters are found, return False.
return False
# Test the 'test' function with different input strings.
str1 = "PHP"
print("Original string: ", str1)
print("Check for consecutive similar letters! ", test(str1))
str2 = "PHHP"
print("\nOriginal string: ", str2)
print("Check for consecutive similar letters! ", test(str2))
str3 = "PHPP"
print("\nOriginal string: ", str3)
print("Check for consecutive similar letters! ", test(str3))
Sample Output:
Original string: PHP Check for consecutive similar letters! False Original string: PHHP Check for consecutive similar letters! True Original string: PHPP Check for consecutive similar letters! True
Explanation:
Here is a breakdown of the above Python code:
- Test Function (test function):
- The "test()" function is defined to check if there are consecutive similar letters in a given string.
- It iterates through each character in the input string and checks if the character repeated twice is present in the string.
Flowchart:
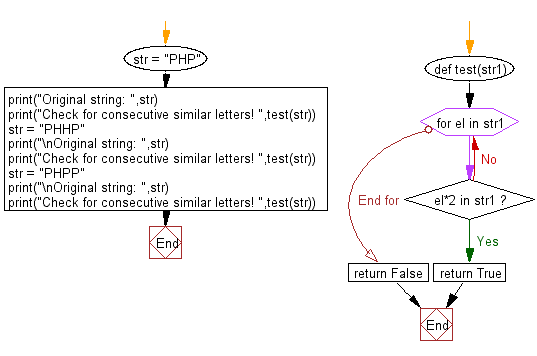
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to remove the first and last elements from a given string.
Next: Write a Python program to reverse a given string in lower case.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/basic/python-basic-1-exercise-124.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics