Python: Remove all vowels from a given string
Remove Vowels from String
Write a Python program to remove all vowels from a given string.
Sample Solution-1:
Python Code:
# Import the 're' module for regular expressions.
import re
# Define a function 'test' that removes vowels from a given text using regular expressions.
def test(text):
# Use the 're.sub' function to replace vowels (case-insensitive) with an empty string.
return re.sub(r'[aeiou]+', '', text, flags=re.IGNORECASE)
# Provide a sample text "Python".
text = "Python"
# Print the original text.
print("Original string:", text)
# Print the result after removing vowels from the sample text using the 'test' function.
print("After removing all the vowels from the said string: " + test(text))
# Provide another sample text "C Sharp".
text = "C Sharp"
# Print the original text.
print("\nOriginal string:", text)
# Print the result after removing vowels from the sample text using the 'test' function.
print("After removing all the vowels from the said string: " + test(text))
# Provide one more sample text "JavaScript".
text = "JavaScript"
# Print the original text.
print("\nOriginal string:", text)
# Print the result after removing vowels from the sample text using the 'test' function.
print("After removing all the vowels from the said string: " + test(text))
Sample Output:
Original string: Python After removing all the vowels from the said string: Pythn Original string: C Sharp After removing all the vowels from the said string: C Shrp Original string: JavaScript After removing all the vowels from the said string: JvScrpt
Explanation:
Here is a breakdown of the above Python code:
- Import Statement:
- The 're' module is imported for regular expression operations.
- Test Function (test function):
- The "test()" function is defined to remove vowels from a given text using regular expressions (re.sub).
- The regular expression [aeiou]+ matches one or more vowels (case-insensitive), and they are replaced with an empty string.
Flowchart:
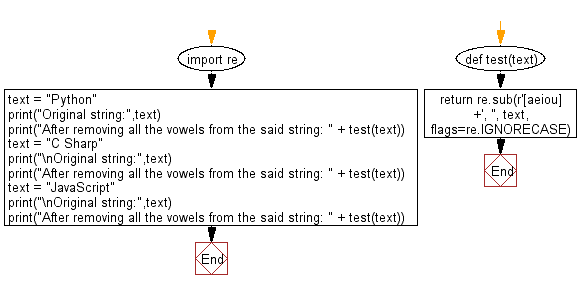
Sample Solution-2:
Python Code:
# Define a function 'test' that removes vowels from a given text.
def test(text):
# Define a string containing all vowels (both uppercase and lowercase).
vowels = "AEIOUaeiou"
# Use a list comprehension to create a new string without vowels.
return ''.join(el for el in text if not el in vowels)
# Provide a sample text "Python".
text = "Python"
# Print the original text.
print("Original string:", text)
# Print the result after removing vowels from the sample text using the 'test' function.
print("After removing all the vowels from the said string: " + test(text))
# Provide another sample text "C Sharp".
text = "C Sharp"
# Print the original text.
print("\nOriginal string:", text)
# Print the result after removing vowels from the sample text using the 'test' function.
print("After removing all the vowels from the said string: " + test(text))
# Provide one more sample text "JavaScript".
text = "JavaScript"
# Print the original text.
print("\nOriginal string:", text)
# Print the result after removing vowels from the sample text using the 'test' function.
print("After removing all the vowels from the said string: " + test(text))
Sample Output:
Original string: Python After removing all the vowels from the said string: Pythn Original string: C Sharp After removing all the vowels from the said string: C Shrp Original string: JavaScript After removing all the vowels from the said string: JvScrpt
Explanation:
Here is a breakdown of the above Python code:
- Test Function (test function):
- The "test()" function is defined to remove vowels from a given text.
- A string 'vowels' is defined to contain all vowels (both uppercase and lowercase).
- A list comprehension is used to create a new string without vowels.
Flowchart:
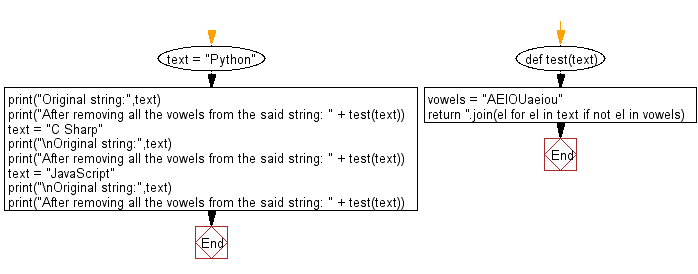
For more Practice: Solve these Related Problems:
- Write a Python program to remove all vowels from a given string and output the resulting text.
- Write a Python program to filter out vowels from an input string using list comprehension.
- Write a Python program to eliminate both uppercase and lowercase vowels from a string.
- Write a Python program to produce a new string by deleting every vowel character from the original string.
Go to:
Previous: Write a Python program to check whether the average value of the elements of a given array of numbers is a whole number or not.
Next: Write a Python program to get the index number of all lower case letters in a given string.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.