Python: Count number of zeros and ones in the binary representation of a given integer
Count Binary Zeros and Ones
Write a Python program to count the number of zeros and ones in the binary representation of a given integer.
Sample Solution
Python Code:
Sample Output:
Original number: 12 Number of ones and zeros in the binary representation of the said number: Number of zeros: 2, Number of ones: 2 Original number: 1234 Number of ones and zeros in the binary representation of the said number: Number of zeros: 6, Number of ones: 5
Explanation:
Here is a breakdown of the above Python code:
- Define Test Function (test function):
- The "test()" function is defined to count the number of ones and zeros in the binary representation of a given number.
- It uses the 'bin' function to get the binary representation of the number as a string.
- The 'replace' method is used to remove the "0b" prefix from the binary representation.
- The 'count' method is used to count the occurrences of '1' and '0'.
- The counts are stored in variables and then formatted into a string for the result.
Flowchart:
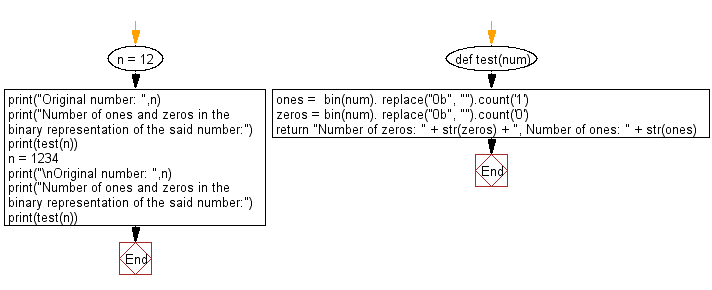
For more Practice: Solve these Related Problems:
- Write a Python program to convert an integer to its binary representation and count the number of zeros and ones.
- Write a Python program to output the counts of zeros and ones in the binary form of a given number.
- Write a Python program to compute and display the number of zeros and ones present in an integer's binary representation.
- Write a Python program to analyze the binary string of an integer and return the counts of each digit (0 and 1).
Go to:
Previous: Write a Python program to check whether a given month and year contains a Monday 13th.
Next: Write a Python program to find all the factors of a given natural number.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.