Python: Reverse the binary representation of an integer and convert it into an integer
Reverse Binary and Convert
Write a Python program to reverse the binary representation of a given number and convert the reversed binary number into an integer.
Sample Solution:
Python Code:
# Define a function 'test' that takes an integer 'n' as input.
def test(n):
# Convert 'n' to binary using 'bin(n)', reverse the binary representation, and remove the '0b' prefix.
reversed_binary = bin(n)[::-1][:-2]
# Convert the reversed binary representation back to an integer using 'int(reversed_binary, 2)'.
return int(reversed_binary, 2)
# Test case 1
n1 = 13
print("Original number: ", n1)
print("Reverse the binary representation of the said integer and convert it into an integer:\n", test(n1))
# Test case 2
n2 = 145
print("\nOriginal number: ", n2)
print("Reverse the binary representation of the said integer and convert it into an integer:\n", test(n2))
# Test case 3
n3 = 1342
print("\nOriginal number: ", n3)
print("Reverse the binary representation of the said integer and convert it into an integer:\n", test(n3))
Sample Output:
Original number: 13 Reverse the binary representation of the said integer and convert it into an integer: 11 Original number: 145 Reverse the binary representation of the said integer and convert it into an integer: 137 Original number: 1342 Reverse the binary representation of the said integer and convert it into an integer: 997
Explanation:
Here is a breakdown of the above Python code:
- test Function:
- The "test()" function takes an integer 'n' as input.
- It converts 'n' to its binary representation using bin(n).
- The binary representation is reversed using [::-1] and the prefix '0b' is removed by [:-2].
- The reversed binary representation is then converted back to an integer using int(reversed_binary, 2).
Flowchart:
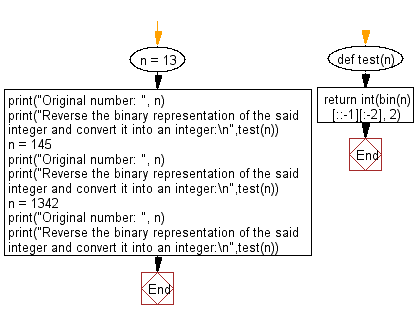
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the longest common ending between two given strings.
Next: Write a Python program to find the closest palindrome number of a given integer. If there are two palindrome numbers in absolute distance return the smaller number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics