Python: Get the domain name using PTR DNS records from a given IP address
Python Basic - 1: Exercise-141 with Solution
Write a Python program to get the domain name using PTR DNS records from a given IP address.
What is a DNS PTR record?
The Domain Name System, or DNS, correlates domain names with IP addresses. A DNS pointer record (PTR for short) provides the domain name associated with an IP address. A DNS PTR record is exactly the opposite of the 'A' record, which provides the IP address associated with a domain name.
DNS PTR records are used in reverse DNS lookups. When a user attempts to reach a domain name in their browser, a DNS lookup occurs, matching the domain name to the IP address. A reverse DNS lookup is the opposite of this process: it is a query that starts with the IP address and looks up the domain name. Source: cloudflare.com
Sample Solution-1:
Python Code:
# Define a function 'get_domain_name' that takes an IP address as an argument.
def get_domain_name(ip_address):
# Import the 'socket' module to perform DNS queries.
import socket
# Use 'socket.gethostbyaddr' to get the domain name associated with the given IP address.
result = socket.gethostbyaddr(ip_address)
# Convert the result to a list and return the first element, which is the domain name.
return list(result)[0]
# Display a message indicating the next section.
print("Domain name using PTR DNS:")
# Call the 'get_domain_name' function with different IP addresses and print the results.
print(get_domain_name("8.8.8.8"))
print(get_domain_name("13.251.106.90"))
print(get_domain_name("8.8.4.4"))
print(get_domain_name("23.23.212.126"))
Sample Output:
Domain name using PTR DNS: dns.google ec2-13-251-106-90.ap-southeast-1.compute.amazonaws.com dns.google ec2-23-23-212-126.compute-1.amazonaws.com
Explanation:
Here is a breakdown of the above Python code:
- get_domain_name Function:
- Defines a function "get_domain_name()" that takes an IP address as an argument.
- Importing the 'socket' Module:
- Imports the "socket" module to perform DNS queries.
- Performing DNS Query:
- Uses socket.gethostbyaddr to get the domain name associated with the given IP address.
- Returning the Domain Name:
- Converts the result to a list and returns the first element, which is the domain name.
- Calling the Function:
- Calls the "get_domain_name()" function with different IP addresses and prints the results.
Flowchart:
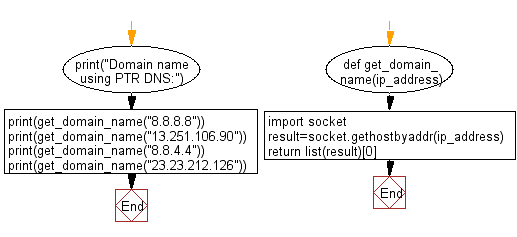
Sample Solution-2:
Python Code:
# Define a function 'get_domain_name' that takes an IP address as an argument.
def get_domain_name(ip_address):
# Import the 'socket' module to perform DNS queries.
import socket
# Use 'socket.getfqdn' to get the fully qualified domain name associated with the given IP address.
return socket.getfqdn(ip_address)
# Display a message indicating the next section.
print("Domain name using PTR DNS:")
# Call the 'get_domain_name' function with different IP addresses and print the results.
print(get_domain_name("8.8.8.8"))
print(get_domain_name("13.251.106.90"))
print(get_domain_name("8.8.4.4"))
print(get_domain_name("23.23.212.126"))
Sample Output:
Domain name using PTR DNS: dns.google ec2-13-251-106-90.ap-southeast-1.compute.amazonaws.com dns.google ec2-23-23-212-126.compute-1.amazonaws.com
Explanation:
Here is a breakdown of the above Python code:
- get_domain_name Function:
- Define a function "get_domain_name()" that takes an IP address as an argument.
- Importing the 'socket' module:
- Import the "socket" module to perform DNS queries.
- Performing DNS query:
- Use socket.getfqdn to get the fully qualified domain name associated with the given IP address.
- Return the domain name:
- Returns the fully qualified domain name obtained from the DNS query.
- Call the function:
- Calls the "get_domain_name()" function with different IP addresses and prints the results.
Flowchart:
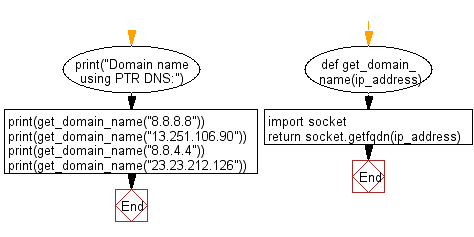
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to convert all items in a given list to float values.
Next: Write a Python program to check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones of same length in a given string. Return True/False.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/basic/python-basic-1-exercise-141.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics