Python: Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones of same length
Python Basic - 1: Exercise-142 with Solution
Write a Python program to check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones of the same length in a given string. Return True/False.
Sample Solution:
Python Code:
# Import the 're' module for regular expressions.
import re
# Define a function 'test' that checks if every consecutive sequence of zeroes is followed by a consecutive sequence of ones.
def test(txt):
# Use regular expressions to find all consecutive sequences of zeroes and ones and compare their lengths.
return [len(i) for i in re.findall('0+', txt)] == [len(i) for i in re.findall('1+', txt)]
# Test the function with different input sequences.
str1 = "001011"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
str1 = "01010101"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
str1 = "00"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
str1 = "000111000111"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
str1 = "00011100011"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
str1 = "0011101"
print("\nOriginal sequence:", str1)
print("Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string:")
print(test(str1))
Sample Output:
Original sequence: 001011 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: False Original sequence: 01010101 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: True Original sequence: 00 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: False Original sequence: 000111000111 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: True Original sequence: 00011100011 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: False Original sequence: 0011101 Check if every consecutive sequence of zeroes is followed by a consecutive sequence of ones in the said string: False
Explanation:
Here is a breakdown of the above Python code:
- Importing the 're' module:
- Import the "re" module for regular expressions.
- Define the 'test' function:
- Define a function "test()" that checks if every consecutive sequence of zeroes is followed by a consecutive sequence of ones.
- Regular expression matching:
- Use regular expressions to find all consecutive sequences of zeroes ('0+') and ones ('1+') in the input string.
- Comparing lengths:
- Compares the lengths of consecutive sequences of zeroes and ones to check the specified condition.
Flowchart:
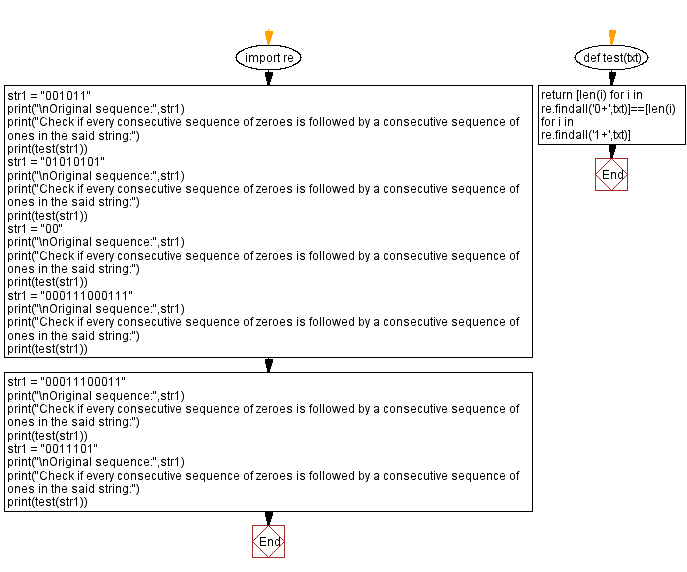
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to get the domain name using PTR DNS records from a given IP address.
Next: Write a Python program to print Emojis using unicode characters or CLDR (Common Locale Data Repository ) short names.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/basic/python-basic-1-exercise-142.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics