Python: Largest and smallest digit of a given number
Largest and Smallest Digits
Write a Python program to find the largest and smallest digits of a given number.
Sample Solution:
Python Code:
# Function to find the largest and smallest digits in a given number.
def Largest_Smallest_digit(n):
# Initialize variables to store the largest and smallest digits.
largest_digit = 0
smallest_digit = 9
# Loop through each digit in the number.
while (n):
# Extract the last digit.
digit = n % 10
# Update the largest digit.
largest_digit = max(digit, largest_digit)
# Update the smallest digit.
smallest_digit = min(digit, smallest_digit)
# Remove the last digit from the number.
n = n // 10
# Return the largest and smallest digits.
return largest_digit, smallest_digit
# Example usage of the function with different numbers.
n = 9387422
print("Original Number:", n)
result = Largest_Smallest_digit(n)
print("Largest Digit of the said number:", result[0])
print("Smallest Digit of the said number:", result[1])
n = 500
print("\nOriginal Number:", n)
result = Largest_Smallest_digit(n)
print("Largest Digit of the said number:", result[0])
print("Smallest Digit of the said number:", result[1])
n = 231548
print("\nOriginal Number:", n)
result = Largest_Smallest_digit(n)
print("Largest Digit of the said number:", result[0])
print("Smallest Digit of the said number:", result[1])
Sample Output:
Original Number: 9387422 Largest Digit of the said number: 9 Smallest Digit of the said number: 2 Original Number: 500 Largest Digit of the said number: 5 Smallest Digit of the said number: 0 Original Number: 231548 Largest Digit of the said number: 8 Smallest Digit of the said number: 1
Explanation:
Here is a breakdown of the above Python code:
- Function Definition:
- def Largest_Smallest_digit(n):: Defines a function named Largest_Smallest_digit that takes an integer 'n' as input.
- Variable Initialization:
- largest_digit = 0: Initializes a variable to store the largest digit, starting with 0.
- smallest_digit = 9: Initializes a variable to store the smallest digit, starting with 9.
- While Loop:
- while (n):: Enters a while loop that continues until the number becomes zero.
- Digit Extraction:
- digit = n % 10: Extracts the last digit of the number.
- Update Largest and Smallest Digits:
- largest_digit = max(digit, largest_digit): Updates the largest digit.
- smallest_digit = min(digit, smallest_digit): Updates the smallest digit.
- Number Modification:
- n = n // 10: Removes the last digit from the number.
- Return Statement:
- return largest_digit, smallest_digit: Returns the largest and smallest digits.
Flowchart:
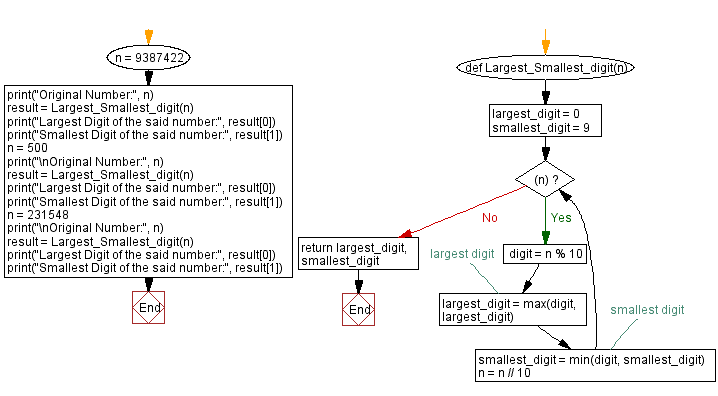
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to convert integer to string.
Next: Check square root and cube root of a number
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics