Python Exercises: Sum of the digits in each number in a list is equal
Python Basic - 1: Exercise-147 with Solution
A Python list contains three positive integers. Write a Python program to check whether the sum of the digits in each number is equal or not. Return true otherwise false.
Sample Data:
([13, 4, 22]) -> True
([-13, 4, 22]) -> False
([45, 63, 90]) -> True
Sample Solution-1:
Python Code:
# Function to check if the sum of digits in each number of the list is equal.
def test(nums):
# Check if the sum of digits in each number of the list is equal.
return nums[0] % 9 == nums[1] % 9 == nums[2] % 9
# Example usage of the function with different lists of numbers.
nums = [13, 4, 22]
print("Original list of numbers:", nums)
print("Check sum of the digits in each number of the said list is equal or not!")
print(test(nums))
nums = [-13, 4, 22]
print("\nOriginal list of numbers:", nums)
print("Check sum of the digits in each number of the said list is equal or not!")
print(test(nums))
nums = [45, 63, 90]
print("\nOriginal list of numbers:", nums)
print("Check sum of the digits in each number of the said list is equal or not!")
print(test(nums))
Sample Output:
Original list of numbers: [13, 4, 22] Check sum of the digits in each number of the said list is equal or not! True Original list of numbers: [-13, 4, 22] Check sum of the digits in each number of the said list is equal or not! False Original list of numbers: [45, 63, 90] Check sum of the digits in each number of the said list is equal or not! True
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- def test(nums):: Defines a function named "test()" that takes a list of three numbers (nums) as input.
- Conditional check:
- return nums[0] % 9 nums[1] % 9 nums[2] % 9: Checks if the sum of the digits in each number of the list is equal.
Flowchart:
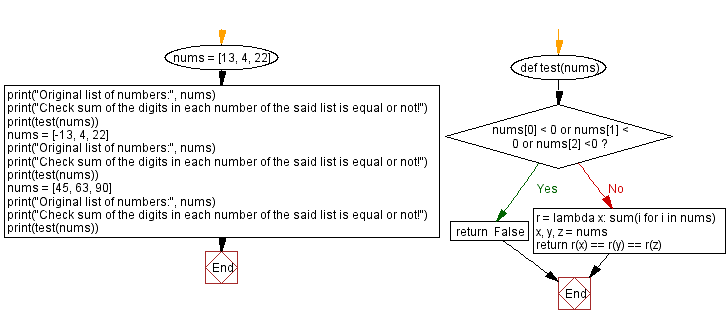
Sample Solution-2:
Python Code:
# Function to check if the sum of digits in each number of the list is equal.
def test(nums):
# Check if any number in the list is negative.
if nums[0] < 0 or nums[1] < 0 or nums[2] < 0:
return False
# Lambda function to calculate the sum of digits in a number.
r = lambda x: sum(int(i) for i in str(x))
# Unpack the list into three variables x, y, and z.
x, y, z = nums
# Check if the sum of digits is equal for all numbers in the list.
return r(x) == r(y) == r(z)
# Example usage of the function with different lists of numbers.
nums = [13, 4, 22]
print("Original list of numbers:", nums)
print("Check sum of the digits in each number of the said list is equal or not!")
print(test(nums))
nums = [-13, 4, 22]
print("\nOriginal list of numbers:", nums)
print("Check sum of the digits in each number of the said list is equal or not!")
print(test(nums))
nums = [45, 63, 90]
print("\nOriginal list of numbers:", nums)
print("Check sum of the digits in each number of the said list is equal or not!")
print(test(nums))
Sample Output:
Original list of numbers: [13, 4, 22] Check sum of the digits in each number of the said list is equal or not! True Original list of numbers: [-13, 4, 22] Check sum of the digits in each number of the said list is equal or not! False Original list of numbers: [45, 63, 90] Check sum of the digits in each number of the said list is equal or not! True
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- def test(nums):: Defines a function named test that takes a list of three numbers (nums) as input.
- Conditional check:
- if nums[0] < 0 or nums[1] < 0 or nums[2] < 0:: Checks if any number in the list is negative.
- return False: Returns False if any number is negative.
- Lambda Function:
- r = lambda x: sum(int(i) for i in str(x)): Defines a lambda function (r) to calculate the sum of digits in a number.
- Unpacking and Comparison:
- x, y, z = nums: Unpacks the list into three variables (x, y, and z).
- return r(x) r(y) r(z): Checks if the sum of digits is equal for all numbers in the list.
Flowchart:
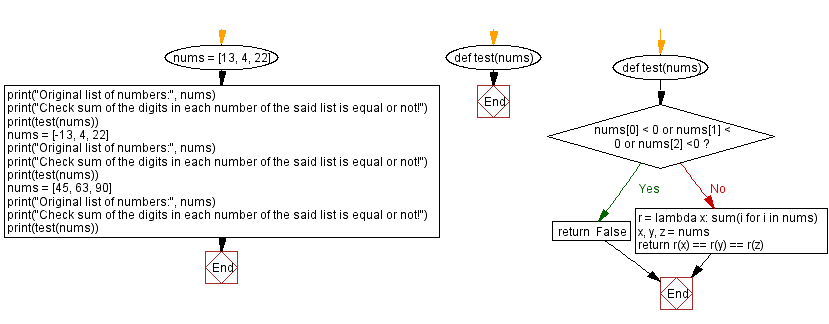
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Check square root and cube root of a number
Next: Check the numbers that are higher than the previous.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/basic/python-basic-1-exercise-147.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics