Python: Find the value of n where n degrees of number 2 are written sequentially in a line without spaces
Python Basic - 1: Exercise-19 with Solution
Write a Python program that finds the value of n when n degrees of number 2 are written sequentially on a line without spaces between them.
Visual Presentation:
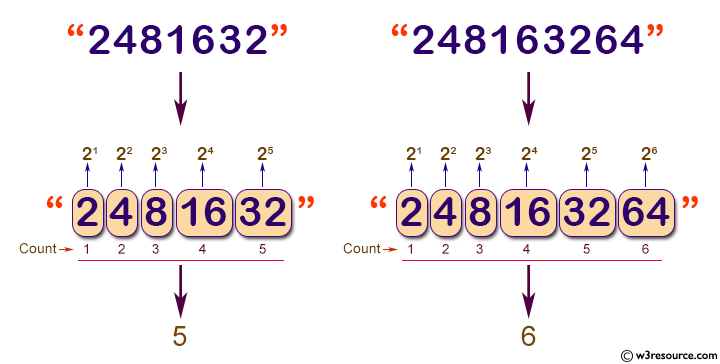
Sample Solution:
Python Code:
# Define a function 'ndegrees' that calculates the highest power of a number 'n'
# such that the resulting number is found within a given input string 'num'.
def ndegrees(num):
ans = True # Initialize a boolean variable 'ans' to True.
n, tempn, i = 2, 2, 2 # Initialize variables 'n', 'tempn', and 'i'.
# Use a while loop to check conditions.
while ans:
# Check if the string representation of 'tempn' is found within 'num'.
if str(tempn) in num:
i += 1 # Increment 'i'.
tempn = pow(n, i) # Update 'tempn' with the next power of 'n'.
else:
ans = False # Set 'ans' to False if 'tempn' is not found in 'num'.
return i - 1 # Return the highest power (i-1).
# Test the 'ndegrees' function with different input strings and print the results.
print(ndegrees("2481632"))
print(ndegrees("248163264"))
Sample Output:
5 6
Explanation:
The above Python code defines a function "ndegrees()" that calculates the highest power of a number 'n' such that the resulting number is found within a given input string 'num'. Here's a brief explanation:
- Function Definition:
- def ndegrees(num):: Define a function named "ndegrees()" that takes a string num as input.
- Initialization:
- ans = True: Initialize a boolean variable 'ans' to 'True'.
- n, tempn, i = 2, 2, 2: Initialize variables 'n' (base number), 'tempn' (temporary result), and i (exponent) to 2.
- While Loop:
- while ans:: Enter a while loop that continues as long as 'ans' is 'True'.
- if str(tempn) in num:: Check if the string representation of 'tempn' is found within the input string 'num'.
- i += 1: Increment the exponent i.
- tempn = pow(n, i): Update 'tempn' with the next power of 'n'.
- else:: If 'tempn' is not found in 'num', set 'ans' to 'False' to exit the loop.
- Return Result:
- return i - 1: Return the highest exponent (i-1) that satisfies the condition.>
- Function Testing:
- print(ndegrees("2481632")): Test the function with the input string "2481632" and print the result.
- print(ndegrees("248163264")): Test the function with the input string "248163264" and print the result.
Flowchart:
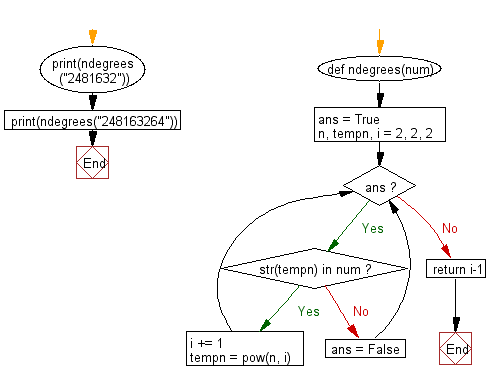
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the median among three given numbers
Next: Write a Python program to find the number of zeros at the end of a factorial of a given positive number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/basic/python-basic-1-exercise-19.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics