Python: Find the number of zeros at the end of a factorial of a given positive number
Factorial Trailing Zeros
Write a Python program to find the number of zeros at the end of a factorial of a given positive number.
Range of the number(n): (1 ≤ n ≤ 2*109).
Sample Solution:
Python Code:
# Define a function 'factendzero' that calculates the number of trailing zeros
# in the factorial of a given number 'n'.
def factendzero(n):
# Initialize variables 'x' and 'y'.
x = n // 5
y = x
# Use a while loop to iteratively calculate the number of trailing zeros.
while x > 0:
x /= 5
y += int(x)
return y # Return the final count of trailing zeros.
# Test the 'factendzero' function with different values of 'n' and print the results.
print(factendzero(5))
print(factendzero(12))
print(factendzero(100))
Sample Output:
1 2 24
Explanation:
The above Python code defines a function "factendzero()" that calculates the number of trailing zeros in the factorial of a given number 'n'. Here's a brief explanation:
- Function Definition:
- def factendzero(n):: Define a function named "factendzero" that takes an integer 'n' as input.
- Variable Initialization:
- x = n // 5: Initialize a variable 'x' to the integer division of 'n' by 5.
- y = x: Initialize another variable 'y' with the same value as 'x'.
- While Loop:
- while x > 0:: Enter a while loop that continues as long as 'x' is greater than 0.
- x /= 5: Divide 'x' by 5 in each iteration.
- y += int(x): Add the integer value of 'x' to 'y'.
- Return Result:
- return y: Return the final count of trailing zeros.
- Function Testing:
- print(factendzero(5)): Test the function with the input 5 and print the result.
- print(factendzero(12)): Test the function with the input 12 and print the result.
- print(factendzero(100)): Test the function with the input 100 and print the result.
Visual Presentation:
Flowchart:
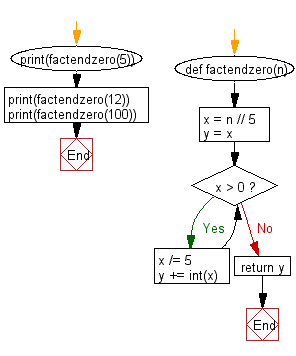
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the value of n where n degrees of number 2 are written sequentially in a line without spaces.
Next: Write a Python program to find the number of notes (Sample of notes: 10, 20, 50, 100, 200 and 500 ) against an given amount.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics