Python: Find the digits which are absent in a given mobile number
Missing Digits Finder
Write a Python program to find the digits that are missing from a given mobile number.
Sample Solution:
Python Code:
# Define a function 'absent_digits' that finds the absent digits from the given list 'n'.
def absent_digits(n):
# Create a set containing all possible digits (0 to 9).
all_nums = set([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
# Convert the list 'n' to a set of integers.
n = set([int(i) for i in n])
# Find the symmetric difference between the set of all_nums and the set 'n'.
n = n.symmetric_difference(all_nums)
# Sort the result to get a list of absent digits.
n = sorted(n)
# Return the list of absent digits.
return n
# Test the 'absent_digits' function with a given list and print the result.
print(absent_digits([9, 8, 3, 2, 2, 0, 9, 7, 6, 3]))
Sample Output:
[1, 4, 5]
Explanation:
The above Python code defines a function named "absent_digits()" that takes a list 'n' as input and finds the digits that are absent from the list. Here's a brief explanation:
- Function Definition:
- def absent_digits(n):: Define a function named "absent_digits()" that takes a list of digits 'n' as input.
- Set of All Possible Digits:
- all_nums = set([0, 1, 2, 3, 4, 5, 6, 7, 8, 9]): Create a set containing all possible digits from 0 to 9.
- Convert List to Set:
- n = set([int(i) for i in n]): Convert the input list 'n' to a set of integers.
- Symmetric Difference:
- n = n.symmetric_difference(all_nums): Find the symmetric difference between the set of all possible digits and the set 'n'. This gives the set of absent digits.
- Sort Result:
- n = sorted(n): Sort the set of absent digits to obtain a list of absent digits.
- Return Result:
- return n: Return the list of absent digits.
- Function Testing:
- print(absent_digits([9, 8, 3, 2, 2, 0, 9, 7, 6, 3])): Test the "absent_digits()" function with a specific list and print the result.
Visual Presentation:
Flowchart:
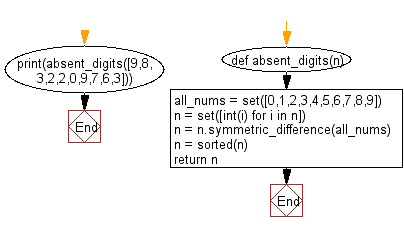
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous:Write a Python program to find the number of divisors of a given integer is even or odd.
Next: Write a Python program to compute the summation of the absolute difference of all distinct pairs in an given array (non-decreasing order).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics