Python: Find common divisors between two numbers in a given pair
Python Basic - 1: Exercise-29 with Solution
Write a Python program to find common divisors between two numbers in a given pair.
Visual Presentation:
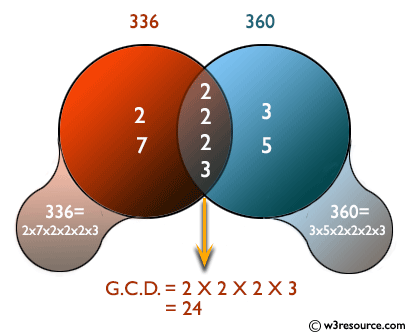
Sample Solution:
Python Code:
# Function to calculate the greatest common divisor (GCD) of two numbers
def ngcd(x, y):
i = 1
while(i <= x and i <= y):
# Check if both x and y are divisible by i
if(x % i == 0 and y % i == 0):
gcd = i # Set gcd to the current common factor
i += 1
return gcd
# Function to calculate the number of common divisors of two numbers
def num_comm_div(x, y):
# Calculate the greatest common divisor (GCD) using the ngcd function
n = ngcd(x, y)
result = 0
z = int(n ** 0.5) # Square root of n
i = 1
while(i <= z):
if(n % i == 0):
result += 2 # Increment the count for each pair of divisors
if(i == n/i):
result -= 1 # Adjust count if i is a perfect square divisor
i += 1
return result
# Test cases
print("Number of common divisors: ", num_comm_div(2, 4))
print("Number of common divisors: ", num_comm_div(2, 8))
print("Number of common divisors: ", num_comm_div(12, 24))
Sample Output:
Number of common divisors: 2 Number of common divisors: 2 Number of common divisors: 6
Explanation:
Here is the breakdown of the above Python exercise:
- User Input:
- tn = int(input("Input third term of the series:")): Takes user input for the third term of the arithmetic series.
- tltn = int(input("Input 3rd last term:")): Takes user input for the third-last term of the arithmetic series.
- s_sum = int(input("Sum of the series:")): Takes user input for the sum of the arithmetic series.
- Calculate Series Length:
- n = int(2 * s_sum / (tn + tltn)): Calculates the length of the arithmetic series using the sum formula.
- Calculate Common Difference:
- Determines the common difference 'd' based on the length of the series.
- If n - 5 == 0, it sets d = (s_sum - 3 * tn) // 6.
- Otherwise, it sets d = (tltn - tn) / (n - 5).
- Calculate First Term:
- a = tn - 2 * d: Calculates the first term 'a' of the arithmetic series using the third term and common difference.
- Print Series:
- Prints the arithmetic series using a loop and the calculated first term and common difference.
Flowchart:
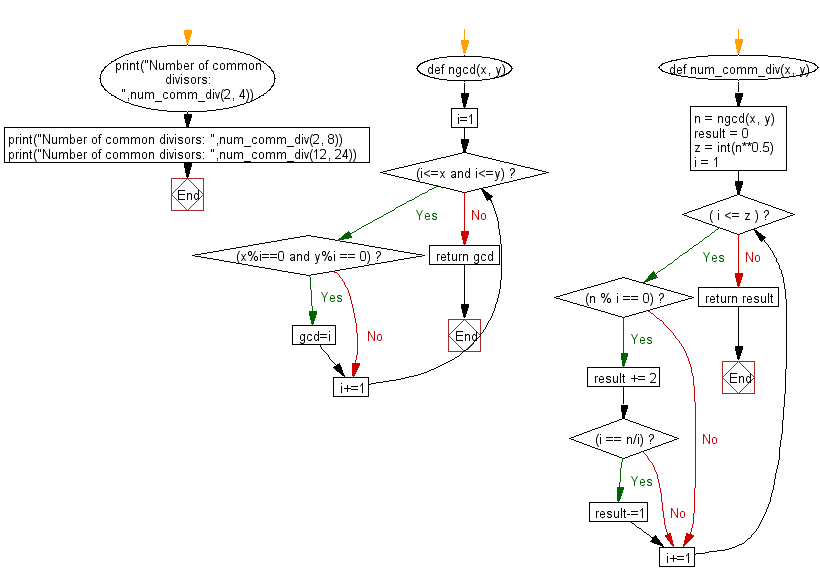
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to print the length of the series and the series from the given 3rd term , 3rd last term and the sum of a series.
Next: Write a Python program to reverse the digits of a given number and add it to the original, If the sum is not a palindrome repeat this procedure.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/basic/python-basic-1-exercise-29.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics