Python: Remove and print every third number from a list of numbers until the list becomes empty
Python Basic - 1: Exercise-3 with Solution
Write a Python program that removes and prints every third number from a list of numbers until the list is empty.
Visual Presentation:
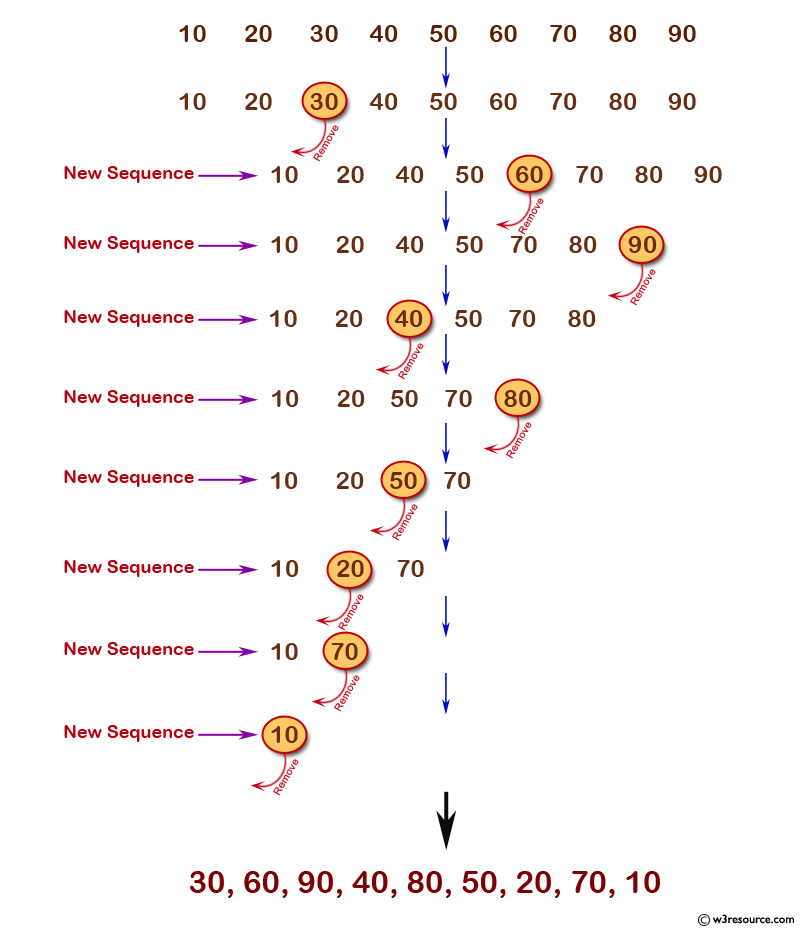
Sample Solution:
Python Code :
# Define a function named 'remove_nums' that takes a list 'int_list' as a parameter.
def remove_nums(int_list):
# Set the starting position for removal to the 3rd element (0-based index).
position = 3 - 1
# Initialize the index variable to 0.
idx = 0
# Get the length of the input list.
len_list = len(int_list)
# Continue removing elements until the list is empty.
while len_list > 0:
# Calculate the new index based on the starting position and current index.
idx = (position + idx) % len_list
# Print and remove the element at the calculated index.
print(int_list.pop(idx))
# Decrement the length of the list.
len_list -= 1
# Create a list of numbers.
nums = [10, 20, 30, 40, 50, 60, 70, 80, 90]
# Call the 'remove_nums' function with the list of numbers.
remove_nums(nums)
Sample Output:
30 60 90 40 80 50 20 70 10
Explanation:
The above code defines a function "remove_nums()" that takes a list as input. It removes and prints elements from the list in a specific pattern until the list is empty. The pattern starts from the 3rd element and removes every 3rd element thereafter. The code then calls this function with a list of numbers and prints the removed elements.
Flowchart:
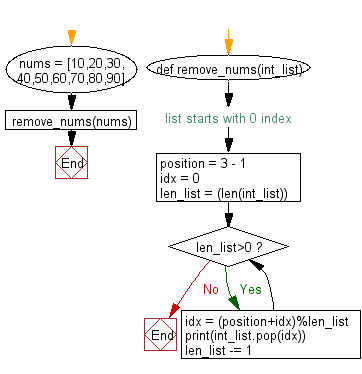
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to create all possible strings by using 'a', 'e', 'i', 'o', 'u'. Use the characters exactly once.
Next: Write a Python program to find unique triplets whose three elements gives the sum of zero from an array of n integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/basic/python-basic-1-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics