Python: Reverse the digits of a given number and add it to the original
Palindrome Sum Iteration
Write a Python program to reverse the digits of a given number and add them to the original. Repeat this procedure if the sum is not a palindrome.
Note: A palindrome is a word, number, or other sequence of characters which reads the same backward as forward, such as madam or racecar.
Visual Presentation:
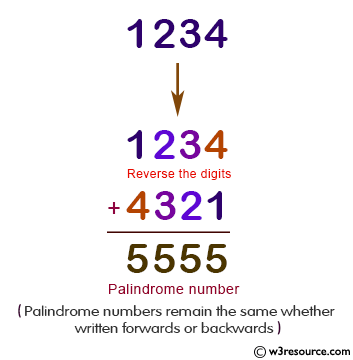
Sample Solution:
Python Code:
# Function to reverse a number and add it to the original number until a palindrome is obtained
def rev_number(n):
s = 0 # Initialize a variable to count the number of iterations
while True:
k = str(n) # Convert the number to a string for comparison
if k == k[::-1]: # Check if the number is a palindrome
break # If it is a palindrome, exit the loop
else:
m = int(k[::-1]) # Reverse the digits and convert back to an integer
n += m # Add the reversed number to the original number
s += 1 # Increment the iteration count
return n # Return the palindrome obtained
# Test cases
print(rev_number(1234)) # Example: 1234 + 4321 = 5555 (palindrome)
print(rev_number(1473)) # Example: 1473 + 3741 = 5214 + 4125 = 9339 (palindrome)
Sample Output:
5555 9339
Explanation:
Here is the breakdown of the above Python exercise:
- Function Definition: The function "rev_number()" takes an integer 'n' as input.
- Initialization: Initializes a variable 's' to count the number of iterations.
- Palindrome Check and Transformation:
- Enters a loop that continues until the number 'n' becomes a palindrome.
- Converts the current number 'n' to a string 'k'.
- Checks if 'k' is equal to its reverse (k[::-1]), indicating a palindrome.
- If not a palindrome, reverse the digits of n (m) and adds it to 'n'.
- Increments in the iteration count 's'.
- Return Palindrome: Returns the final palindrome obtained.
- Test Cases:
- Calls the rev_number function with different initial numbers to demonstrate the transformation into palindromes.
- Example: rev_number(1234) results in 5555 because 1234 + 4321 = 5555, which is a palindrome.
- Example: rev_number(1473) results in 9339 after multiple iterations, forming a palindrome.
Flowchart:
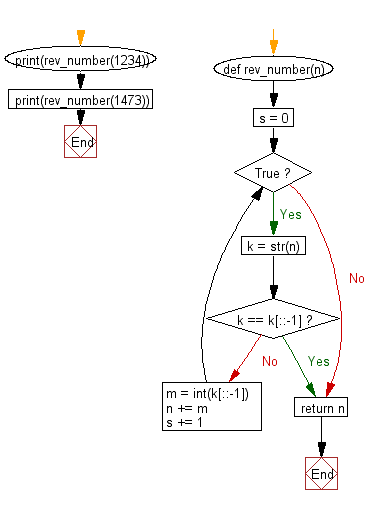
For more Practice: Solve these Related Problems:
- Write a Python program to multiply a number by its reverse repeatedly until the product is a palindrome.
- Write a Python program to subtract the reverse of a number from the number repeatedly until a palindrome is reached.
- Write a Python program to convert a number to a string and check for palindrome status, iterating an operation until it becomes palindromic.
- Write a Python program to determine the number of iterations required for a given number to become a palindrome when adding its reverse.
Go to:
Previous: Write a Python program to print the length of the series and the series from the given 3rd term , 3rd last term and the sum of a series.
Next: Write a Python program to count the number of carry operations for each of a set of addition problems.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.