Python: Check whether three given lengths of three sides form a right triangle
Python Basic - 1: Exercise-34 with Solution
Write a Python program to check whether three given lengths (integers) of three sides form a right triangle. Print "Yes" if the given sides form a right triangle otherwise print "No".
Input:
Integers separated by a single space.
1 ≤ length of the side ≤ 1,000
Visual Presentation:
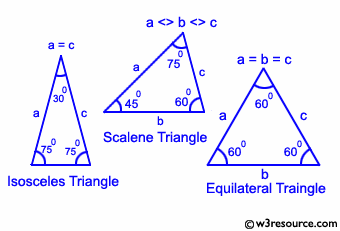
Sample Solution:
Python Code:
# Print statement to prompt the user to input three integers representing sides of a triangle
print("Input three integers (sides of a triangle)")
# Using map and split to take three space-separated integers as input, then converting them to a list of integers
int_num = list(map(int, input().split()))
# Sorting the list of integers to assign variables x, y, and z with the values of the sorted sides
x, y, z = sorted(int_num)
# Checking if the given sides form a Pythagorean triple (right-angled triangle)
if x**2 + y**2 == z**2:
print('Yes') # Print 'Yes' if the condition is met
else:
print('No') # Print 'No' if the condition is not met
Sample Output:
Input three integers(sides of a triangle) 8 6 7 No
Explanation:
Here is the breakdown of the above Python exercise:
- Prints a prompt asking the user to input three integers representing triangle sides.
- Takes the input as three space-separated integers, converts them to a list of integers.
- Sorts the list by assigning variables x, y, and z with the values of the sorted sides.
- Check whether the sorted sides form a Pythagorean triple (right-angled triangle) using the Pythagorean theorem.
- Prints 'Yes' if the sides satisfy the Pythagorean theorem, otherwise prints 'No'.
Flowchart:
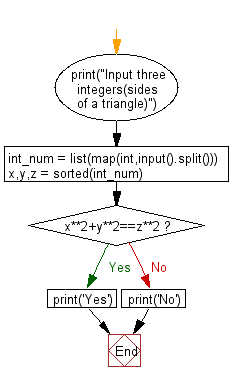
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to compute the digit number of sum of two given integers.
Next: Write a Python program which solve the specified equation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/basic/python-basic-1-exercise-34.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics