Python: Find the longest common prefix string amongst a given array of strings
Longest Common Prefix
Write a Python program to find the longest common prefix string among a given array of strings. Return false if there is no common prefix.
For Example, longest common prefix of "abcdefgh" and "abcefgh" is "abc".
Sample Solution:
Python Code:
# Function to find the longest common prefix among a list of strings
def longest_Common_Prefix(str1):
if not str1: # Check if the list is empty
return ""
short_str = min(str1, key=len) # Find the shortest string in the list
for i, char in enumerate(short_str): # Iterate through characters in the shortest string
for other in str1: # Iterate through other strings in the list
if other[i] != char: # Check if the corresponding characters don't match
return short_str[:i] # Return the common prefix found so far
return short_str # Return the entire shortest string if it is a common prefix
# Test cases
print(longest_Common_Prefix(["abcdefgh", "abcefgh"])) # "abc"
print(longest_Common_Prefix(["w3r", "w3resource"])) # "w3r"
print(longest_Common_Prefix(["Python", "PHP", "Perl"])) # ""
print(longest_Common_Prefix(["Python", "PHP", "Java"])) # ""
Sample Output:
abc w3r P
Explanation:
Here is a breakdown of the above Python code:
- First define a function named "longest_Common_Prefix()" that takes a list of strings (str1) as input.
- Check if the list is empty. If so, return an empty string.
- Find the shortest string (short_str) in the list using the "min()" function with the key=len argument.
- Iterate through the characters in the shortest string using 'enumerate'.
- For each character, iterate through the other strings in the list and check if the corresponding characters match.
- If a mismatch is found, return the common prefix found so far.
- If no mismatch is found, return the entire shortest string as the common prefix.
- Test the function with various input lists and print the results.
Visual Presentation:
Flowchart:
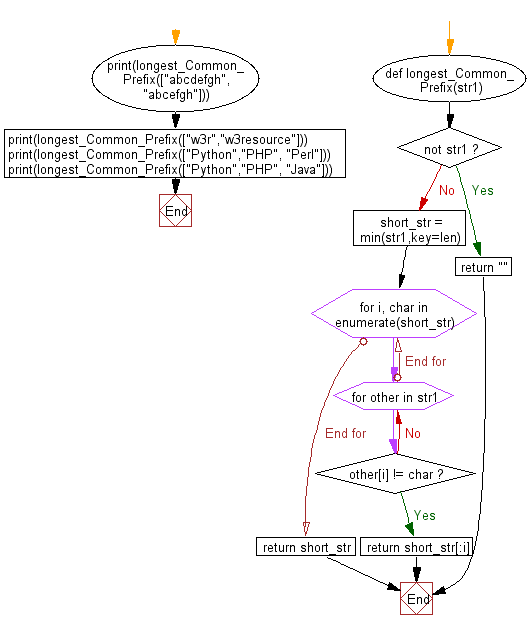
For more Practice: Solve these Related Problems:
- Write a Python program to find the longest common prefix among an array of strings using vertical scanning.
- Write a Python program to compute the longest common prefix using horizontal scanning of string characters.
- Write a Python program to determine the longest common prefix by sorting the list and comparing the first and last elements.
- Write a Python program to implement a divide and conquer approach to find the longest common prefix in a list of strings.
Go to:
Previous: Write a Python program to check if two given strings are isomorphic to each other or not.
Next: Write a Python program to reverse only the vowels of a given string.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.