Python: Find the starting and ending position of a given value in a given array of integers, sorted in ascending order
Find Value Range in Array
Write a Python program to find the starting and ending position of a given value in an array of integers, sorted in ascending order.
If the target is not found in the array, return [-1, 0].
Input: [5, 7, 7, 8, 8, 8] target value = 8
Output: [3, 5]
Input: [1, 3, 6, 9, 13, 14] target value = 4
Output: [-1, 0]
Sample Solution:
Python Code:
# Define a function to find the range of indices for a target value in a sorted list
def search_Range(array_nums, target_val):
# Initialize an empty list to store the result
result_arra = []
# Initialize variables for start and end positions
start_pos = -1
end_pos = 0
# Iterate through the list
for i in range(len(array_nums)):
# Check if the current element is equal to the target value and start_pos is -1
if target_val == array_nums[i] and start_pos == -1:
# Set start_pos to the current index
start_pos = i
# Set end_pos to the current index
end_pos = i
# Check if the current element is equal to the target value and start_pos is not -1
elif target_val == array_nums[i] and start_pos != -1:
# Update end_pos to the current index
end_pos = i
# Append start_pos and end_pos to the result list
result_arra.append(start_pos)
result_arra.append(end_pos)
# Return the result list
return result_arra
# Test the function with different lists and target values, and print the results
print(search_Range([5, 7, 7, 8, 8, 8], 8))
print(search_Range([1, 3, 6, 9, 13, 14], 4))
print(search_Range([5, 7, 7, 8, 10], 8))
Sample Output:
[3, 5] [-1, 0] [3, 3]
Explanation:
Here is a breakdown of the above Python code:
- The function "search_Range()" finds the range of indices for a target value in a sorted list.
- It initializes an empty list 'result_arra' to store the result.
- It initializes variables 'start_pos' and 'end_pos'.
- It iterates through the list using a for loop.
- If the current element is equal to the target value and 'start_pos' is -1, it sets 'start_pos' and 'end_pos' to the current index.
- If the current element is equal to the target value and 'start_pos' is not -1, it updates 'end_pos' to the current index.
- It appends 'start_pos' and 'end_pos' to the result list.
- It returns the result list.
- Test the function with different lists and target values, and print the results.
Flowchart:
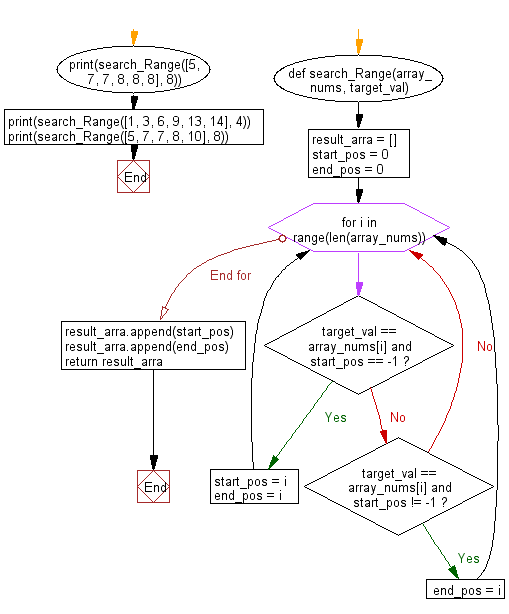
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to remove all instances of a given value from a given array of integers and find the length of the new array.
Next: Write a Python program to find the maximum profit in one transaction.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics