Python: Randomly generate a list with 10 even numbers between 1 and 100 inclusive
Python Basic - 1: Exercise-81 with Solution
Write a Python program to randomly generate a list of 10 even numbers between 1 and 100 inclusive.
Note: Use random.sample() to generate a list of random values.
Sample Solution:
Python Code:
# Import the 'random' module to generate random numbers
import random
# Print a sample of 10 random even numbers from the range 1 to 99
print(random.sample([i for i in range(1, 100) if i % 2 == 0], 10))
Sample Output:
[4, 22, 8, 20, 24, 12, 30, 98, 28, 48]
Explanation:
Here is a breakdown of the above Python code:
- The "random" module is imported to facilitate the generation of random numbers.
- A list comprehension is used to create a list of even numbers in the range from 1 to 99.
- The "random.sample()" function is employed to obtain a random sample of 10 elements from the generated list.
- The result is printed, showcasing a sample of 10 random even numbers from the specified range.
Pictorial Presentation:
Flowchart:
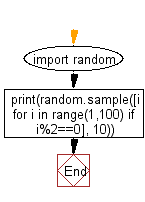
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find the first missing positive integer that does not exist in a given list.
Next: Write a Python program to calculate the median from a list of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/basic/python-basic-1-exercise-81.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics