Python: Count the number of arguments in a given function
Python Basic - 1: Exercise-91 with Solution
Write a Python program to count the number of arguments in a given function.
Sample Solution:
Python Code:
# Define a function named num_of_args that takes a variable number of arguments (*args).
def num_of_args(*args):
# Return the number of arguments passed to the function using the len() function.
return(len(args))
# Test the function with different numbers of arguments and print the results.
# Test case 1: No arguments
print(num_of_args()) # Expecting 0
# Test case 2: One argument
print(num_of_args(1)) # Expecting 1
# Test case 3: Two arguments
print(num_of_args(1, 2)) # Expecting 2
# Test case 4: Three arguments
print(num_of_args(1, 2, 3)) # Expecting 3
# Test case 5: Four arguments
print(num_of_args(1, 2, 3, 4)) # Expecting 4
# Test case 6: One argument (a list)
print(num_of_args([1, 2, 3, 4])) # Expecting 1 (the list is considered a single argument)
Sample Output:
0 1 2 3 4 1
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "num_of_args()" that takes a variable number of arguments (*args).
- Return Statement:
- The function returns the number of arguments passed to it using the "len()" function.
- Test cases:
- The function is tested with different numbers and types of arguments using print(num_of_args(...)).
Visual Presentation:
Flowchart:
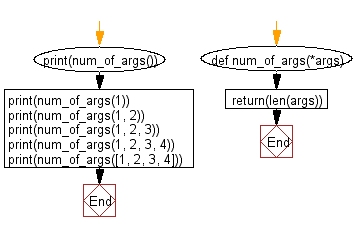
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to replace all but the last five characters of a given string with "*" and returns the new string.
Next: Write a Python program to compute cumulative sum of numbers of a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/basic/python-basic-1-exercise-91.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics