Python: Find the middle character(s) of a given string
Find Middle Character(s)
Write a Python program to find the central character(s) of a given string. Return the two middle characters if the length of the string is even. Return the middle character if the length of the string is odd.
Sample Solution:
Python Code:
# Define a function named middle_char that takes a string (txt) as an argument.
def middle_char(txt):
# Return the middle character(s) of the input string.
# The slicing expression [(len(txt)-1)//2:(len(txt)+2)//2] extracts the middle character(s) for both odd and even-length strings.
return txt[(len(txt)-1)//2:(len(txt)+2)//2]
# Test the function with different strings and print the results.
# Test case 1
text = "Python"
# Print the original string.
print("Original string: ", text)
# Print the middle character(s) of the string.
print("Middle character(s) of the said string: ", middle_char(text))
# Test case 2
text = "PHP"
# Print the original string.
print("\nOriginal string: ", text)
# Print the middle character(s) of the string.
print("Middle character(s) of the said string: ", middle_char(text))
# Test case 3
text = "Java"
# Print the original string.
print("\nOriginal string: ", text)
# Print the middle character(s) of the string.
print("Middle character(s) of the said string: ", middle_char(text))
Output:
Original string: Python Middle character(s) of the said string: th Original string: PHP Middle character(s) of the said string: H Original string: Java Middle character(s) of the said string: av
Explanation:
Here is a breakdown of the above Python code:
- Function definition:
- The code defines a function named "middle_char()" that takes a string (txt) as an argument.
- Slicing Expression:
- The function uses a slicing expression [(len(txt)-1)//2:(len(txt)+2)//2] to extract the middle character(s) of the input string.
- Middle character calculation:
- For odd-length strings, the slicing extracts a single middle character. For even-length strings, it extracts two middle characters.
Visual Presentation:
Flowchart:
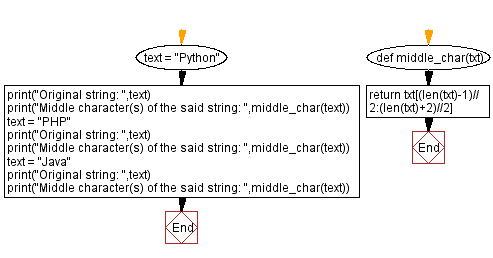
For more Practice: Solve these Related Problems:
- Write a Python program to return the middle character(s) of a given string based on its length.
- Write a Python program to extract the central character(s) from a word, handling both even and odd length cases.
- Write a Python program to determine and print the middle section of a string using precise indexing.
- Write a Python program to calculate the indices for the central character(s) of a string and output the substring.
Go to:
Previous: Write a Python program to compute cumulative sum of numbers of a given list.
Next: Write a Python program to find the largest product of the pair of adjacent elements from a given list of integers.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.