Python Bisect: Insert items into a list in sorted order
3. Sorted Insert into List
Write a Python program to insert items into a list in sorted order.
Sample Solution:
Python Code:
import bisect
# Sample list
my_list = [25, 45, 36, 47, 69, 48, 68, 78, 14, 36]
print("Original List:")
print(my_list)
sorted_list = []
for i in my_list:
position = bisect.bisect(sorted_list, i)
bisect.insort(sorted_list, i)
print("Sorted List:")
print(sorted_list)
Sample Output:
Original List: [25, 45, 36, 47, 69, 48, 68, 78, 14, 36] Sorted List: [14, 25, 36, 36, 45, 47, 48, 68, 69, 78]
Flowchart:
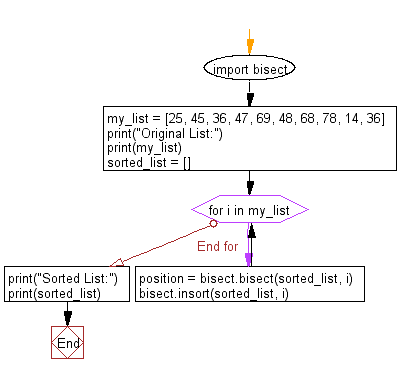
For more Practice: Solve these Related Problems:
- Write a Python program to insert an element into a sorted list while maintaining order, using the bisect module, and then print the updated list.
- Write a Python script to take an unsorted list, sort it, and then insert new elements in their correct sorted position using bisect.insort.
- Write a Python program to implement a function that accepts a list and an item, uses bisect to insert the item, and then verifies the list remains sorted.
- Write a Python function that reads a list of numbers, sorts it, and then sequentially inserts additional values in order using bisect.insort, printing the list after each insertion.
Go to:
Previous: Write a Python program to locate the right insertion point for a specified value in sorted order.
Next: Write a Python program to find the first occurrence of a given number in a sorted list using Binary Search (bisect).Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.