Python Bisect: Find the first occurrence of a given number in a sorted list using Binary Search
Python Bisect: Exercise-4 with Solution
Write a Python program to find the first occurrence of a given number in a sorted list using Binary Search (bisect).
Sample Solution:
Python Code:
from bisect import bisect_left
def Binary_Search(a, x):
i = bisect_left(a, x)
if i != len(a) and a[i] == x:
return i
else:
return -1
nums = [1, 2, 3, 4, 8, 8, 10, 12]
x = 8
num_position = Binary_Search(nums, x)
if num_position == -1:
print(x, "is not present.")
else:
print("First occurrence of", x, "is present at index", num_position)
Sample Output:
First occurrence of 8 is present at index 4
Flowchart:
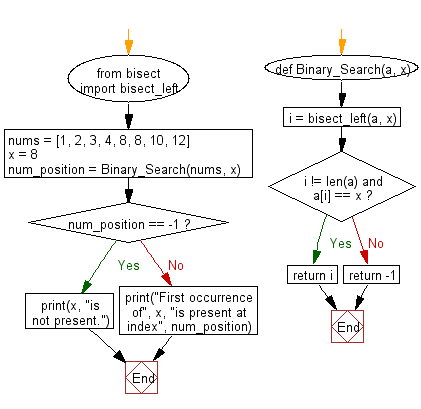
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to insert items into a list in sorted order.
Next: Write a Python program to find the index position of the largest value smaller than a given number in a sorted list using Binary Search (bisect).What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/bisect/python-bisect-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics