Python Bisect: Find the index position of the largest value smaller than a given number in a sorted list using Binary Search
Python Bisect: Exercise-5 with Solution
Write a Python program to find the index position of the largest value smaller than a given number in a sorted list using Binary Search (bisect).
Sample Solution:
Python Code:
from bisect import bisect_left
def Binary_Search(l, x):
i = bisect_left(l, x)
if i:
return (i-1)
else:
return -1
nums = [1, 2, 3, 4, 8, 8, 10, 12]
x = 5
num_position = Binary_Search(nums, x)
if num_position == -1:
print("Not found..!")
else:
print("Largest value smaller than ", x, " is at index ", num_position )
Sample Output:
Largest value smaller than 5 is at index 3
Flowchart:
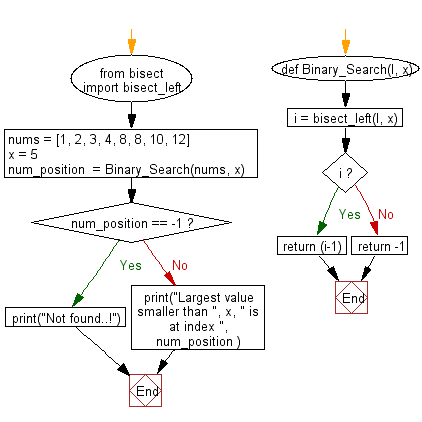
Python Code Editor:
Contribute your code and comments through Disqus.
Next: Write a Python program to find the index position of the last occurrence of a given number in a sorted list using Binary Search (bisect).What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/bisect/python-bisect-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics