Python Challenges: Find the 1000th prime number
Write a python program to find the 1000th prime number.
A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself. By Euclid's theorem, there are an infinite number of prime numbers. Subsets of the prime numbers may be generated with various formulas for primes. The first twenty prime numbers are: 2, 3, 5, 7, 11, 13, 17, 19, 23, 29, 31, 37, 41, 43, 47, 53, 59, 61, 67, 71.
Sample Solution:
Python Code:
def eratosthenes():
D = {}
q = 2
while 1:
if q not in D:
yield q
D[q*q] = [q]
else:
for p in D[q]:
D.setdefault(p+q,[]).append(p)
del D[q]
q += 1
def nth_prime(n):
for i, prime in enumerate(eratosthenes()):
if i == n - 1:
return prime
print(nth_prime(1000))
Sample Output:
7919
Flowchart:
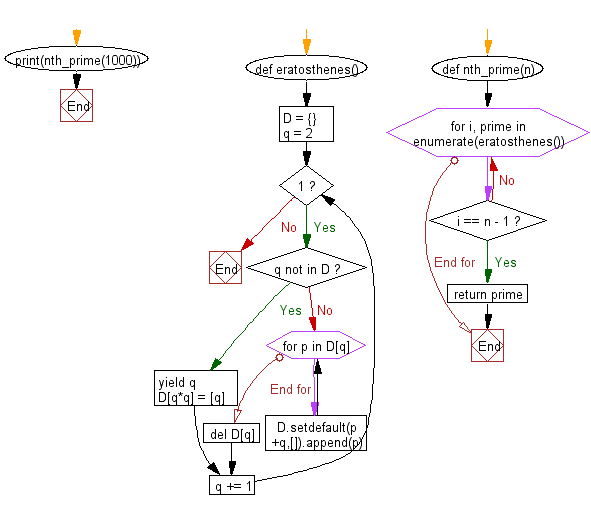
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.