Python Challenges: Find equilibrium index from a given array
Write a Python program to find equilibrium index from a given array. If there is no equilibrium index return 1.
Ref: https://rosettacode.org/wiki/Equilibrium_index
Sample Solution:
Python Code:
def equilibrium(a):
"""https://bit.ly/33Spl45"""
if len(a) == 0:
return -1
left_sum = 0
for i in range(len(a) - 1):
right_sum = 0
middle_point = i + 1
left_sum += a[i]
for j in range(middle_point + 1, len(a)):
right_sum += a[j]
if left_sum == right_sum:
return middle_point
return -1
print(equilibrium([1,4,2,0,3,8,-4]))
print(equilibrium([]))
Sample Output:
3 -1
Flowchart:
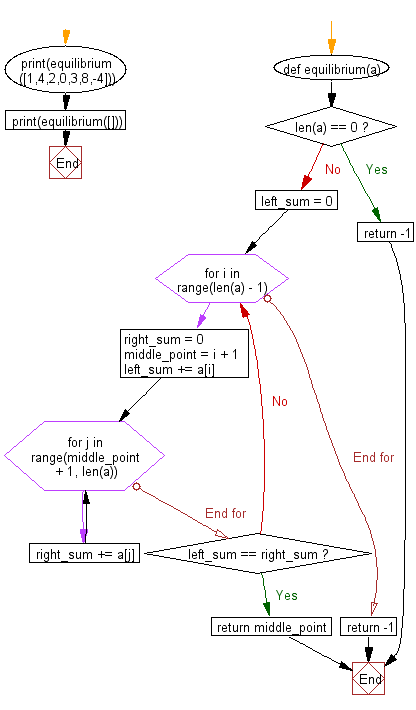
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to compute the edit distance between two given strings.
Next: Write a Python program to find the largest palindrome made from the product of two 4-digit numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.