Python Challenges: Compute the sum of the numbers on the diagonals in a 1001 by 1001 spiral formed
Starting with the number 1 and moving to the right in a clockwise direction a 5 by 5 spiral is formed as follows:
21 |
22 |
23 |
24 |
25 |
20 |
7 |
8 |
9 |
10 |
19 |
6 |
1 |
2 |
11 |
18 |
5 |
4 |
3 |
12 |
17 |
16 |
15 |
14 |
13 |
Diagonals Numbers: 21 + 7 + 1 + 3 + 13 + 25 + 9 + 5 + 17 = 101
It can be verified that the sum of the numbers on the diagonals is 101.
Write a Python program to compute the sum of the numbers on the diagonals in a 1001 by 1001 spiral formed in the above way.
Sample Solution:
Python Code:
MAX_SIZE = 1001
result = 1
result += sum(4 * i * i - 6 * (i - 1) for i in range(3, MAX_SIZE + 1, 2))
print(str(result))
Sample Output:
669171001
Flowchart:
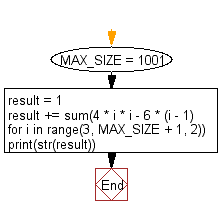
#Ref: https://bit.ly/2GZmz51
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to get a dictionary mapping keys to huffman codes for a frequency table mapping keys to frequencies.
Next: Write a Python program to print a truth table for an infix logical expression.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.