Python Challenges: Calculate sum of all positive, negative integers present in a given string
Write a Python program to calculate sum of all positive, negative integers present in a given string.
Sample Solution-1:
Python Code:
from re import findall
def positive_negative_sum(str1):
return sum(map(int, findall(r'\d+', str1))), sum(map(int, findall(r'-\d+', str1)))
str1 = '-100#^sdfkj8902w3ir021@swf-20'
print("Original string:")
print(str1)
print("\nSum of all positive, negative integers present in the said string:")
result = positive_negative_sum(str1)
print("Positive values:",result[0])
print("Negative values:",result[1])
Sample Output:
Original string: -100#^sdfkj8902w3ir021@swf-20 Sum of all positive, negative integers present in the said string: Positive values: 9046 Negative values: -120
Flowchart:
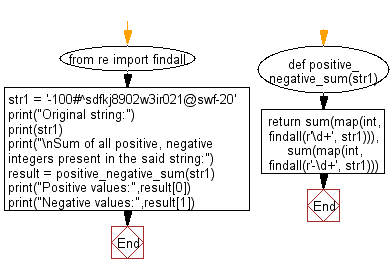
Sample Solution-2:
Python Code:
import re
def positive_negative_sum(str1):
positive_values = sum(int(n) for n in re.findall('\d+', str1))
negative_values = sum(int(n) for n in re.findall('-\d+', str1))
return positive_values, negative_values
str1 = '-100#^sdfkj8902w3ir021@swf-20'
print("Original string:")
print(str1)
print("\nSum of all positive, negative integers present in the said string:")
result = positive_negative_sum(str1)
print("Positive values:",result[0])
print("Negative values:",result[1])
Sample Output:
Original string: -100#^sdfkj8902w3ir021@swf-20 Sum of all positive, negative integers present in the said string: Positive values: 9046 Negative values: -120
Flowchart:
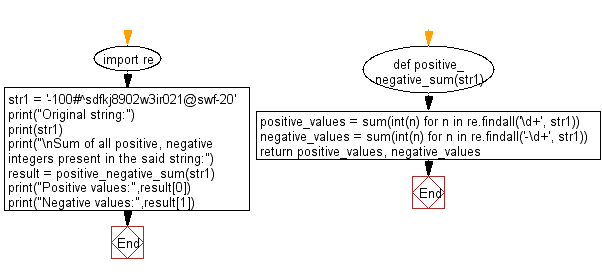
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to reverse and add the numbers of the two linked lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics