Python Challenges: Find three numbers from an array such that the sum of three numbers equal to zero
Write a Python program to find three numbers from an array such that the sum of three numbers equal to zero.
Explanation:
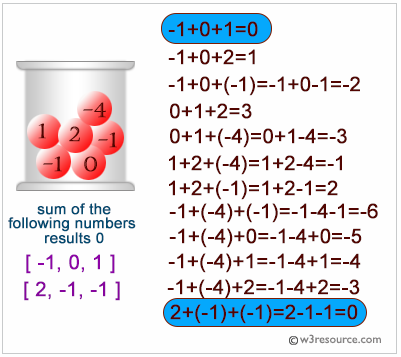
Sample Solution:
Python Code:
# return a list of lists of length 3
def three_Sum(num):
if len(num)<3: return []
num.sort()
result=[]
for i in range(len(num)-2):
left=i+1
right=len(num)-1
if i!=0 and num[i]==num[i-1]:continue
while left<right:
if num[left]+num[right]==-num[i]:
result.append([num[i],num[left],num[right]])
left=left+1
right=right-1
while num[left]==num[left-1] and left<right:left=left+1
while num[right]==num[right+1] and left<right: right=right-1
elif num[left]+num[right]<-num[i]:
left=left+1
else:
right=right-1
return result
nums1=[-1,0,1,2,-1,-4]
nums2 = [-25,-10,-7,-3,2,4,8,10]
print(three_Sum(nums1))
print(three_Sum(nums2))
Sample Output:
[[-1, -1, 2], [-1, 0, 1]] [[-10, 2, 8], [-7, -3, 10]]
Flowchart:
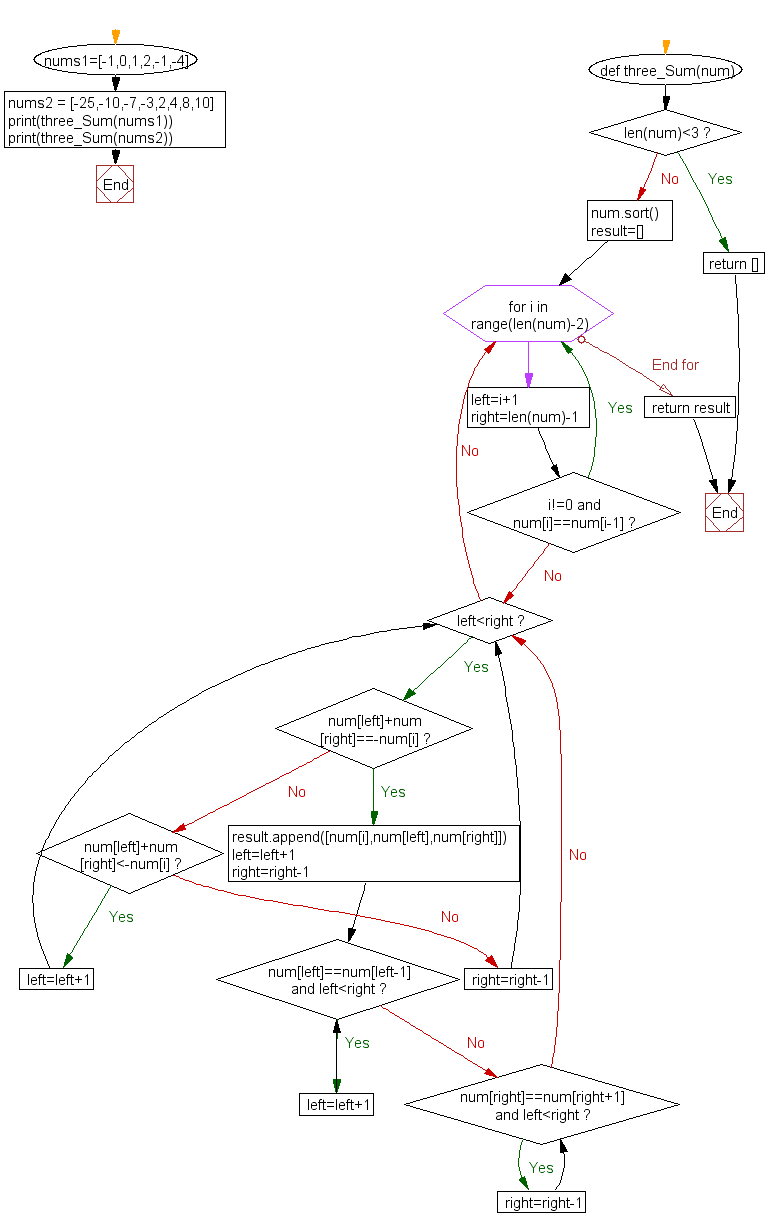
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python program to find missing numbers from a list.
Next: Write a Python program to find three numbers from an array such that the sum of three numbers equal to a given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.