Python: Add and remove attribute in a given class
Write a Python class named Student with two attributes student_id, student_name. Add a new attribute student_class and display the entire attribute and the values of the class. Now remove the student_name attribute and display the entire attribute with values.
Sample Solution:
Python Code:
class Student:
student_id = 'V10'
student_name = 'Jacqueline Barnett'
print("Original attributes and their values of the Student class:")
for attr, value in Student.__dict__.items():
if not attr.startswith('_'):
print(f'{attr} -> {value}')
print("\nAfter adding the student_class, attributes and their values with the said class:")
Student.student_class = 'V'
for attr, value in Student.__dict__.items():
if not attr.startswith('_'):
print(f'{attr} -> {value}')
print("\nAfter removing the student_name, attributes and their values from the said class:")
del Student.student_name
#delattr(Student, 'student_name')
for attr, value in Student.__dict__.items():
if not attr.startswith('_'):
print(f'{attr} -> {value}')
Sample Output:
Original attributes and their values of the Student class: student_id -> V10 student_name -> Jacqueline Barnett After adding the student_class, attributes and their values with the said class: student_id -> V10 student_name -> Jacqueline Barnett student_class -> V After removing the student_name, attributes and their values from the said class: student_id -> V10 student_class -> V
Flowchart:
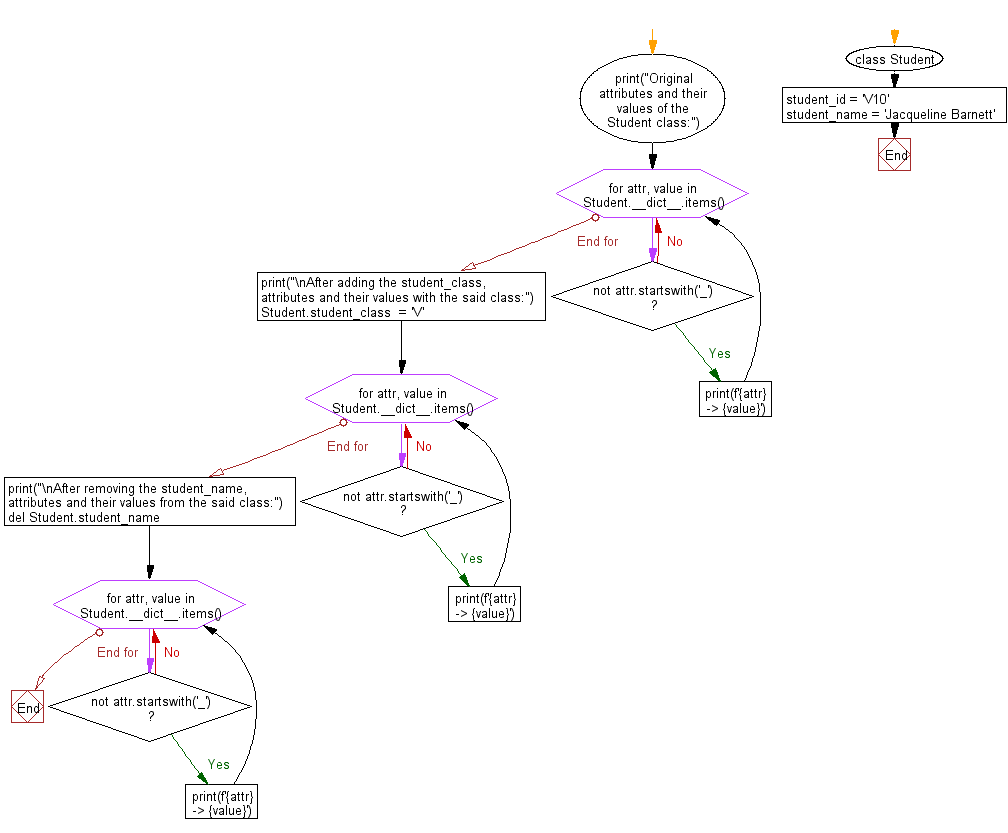
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python class named Student with two attributes student_name, marks. Modify the attribute values of the said class and print the original and modified values of the said attributes.
Next: Write a Python class named Student with two attributes student_id, student_name. Add a new attribute student_class. Create a function to display the entire attribute and their values in Student class.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics