Python: A class constructed by a length and width and a method which will compute the area of a rectangle
10. Class Named Rectangle to Compute Area
Write a Python class named Rectangle constructed from length and width and a method that will compute the area of a rectangle.
Python: Area of a rectangle
In Euclidean plane geometry, a rectangle is a quadrilateral with four right angles. To find the area of a rectangle, multiply the length by the width.
A rectangle with four sides of equal length is a square.
Following image represents the area of a rectangle.
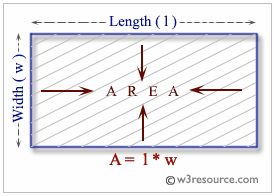
Sample Solution:
Python Code:
class Rectangle():
def __init__(self, l, w):
self.length = l
self.width = w
def rectangle_area(self):
return self.length*self.width
newRectangle = Rectangle(12, 10)
print(newRectangle.rectangle_area())
Sample Output:
120
Flowchart:
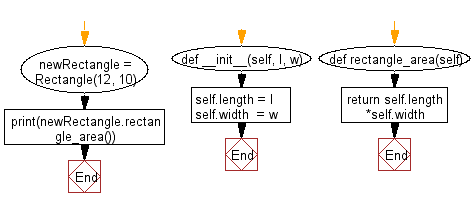
For more Practice: Solve these Related Problems:
- Write a Python class named Rectangle with attributes for length and width and a method to compute and return its area.
- Write a Python class to calculate the perimeter and area of a rectangle, and include input validation for positive dimensions.
- Write a Python class to override the __str__ method to display the rectangle's dimensions and computed area.
- Write a Python class that allows updating rectangle dimensions and recalculates the area dynamically upon change.
Go to:
Previous: Write a Python class which has two methods get_String and print_String. get_String accept a string from the user and print_String print the string in upper case.
Next: Write a Python class named Circle constructed by a radius and two methods which will compute the area and the perimeter of a circle.
Python Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.