Python: Convert a roman numeral to an integer
Python Class: Exercise-2 with Solution
Write a Python class to convert a Roman numeral to an integer.
Sample Solution:
Python Code:
class py_solution:
def roman_to_int(self, s):
rom_val = {'I': 1, 'V': 5, 'X': 10, 'L': 50, 'C': 100, 'D': 500, 'M': 1000}
int_val = 0
for i in range(len(s)):
if i > 0 and rom_val[s[i]] > rom_val[s[i - 1]]:
int_val += rom_val[s[i]] - 2 * rom_val[s[i - 1]]
else:
int_val += rom_val[s[i]]
return int_val
print(py_solution().roman_to_int('MMMCMLXXXVI'))
print(py_solution().roman_to_int('MMMM'))
print(py_solution().roman_to_int('C'))
Sample Output:
3986 4000 100
Pictorial Presentation:
Flowchart:
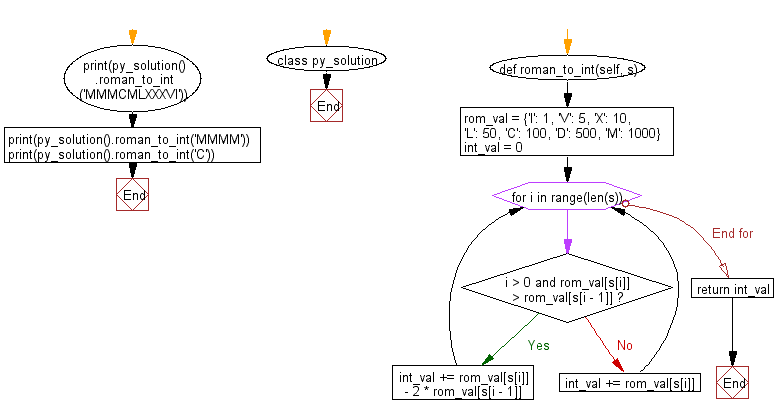
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python class to convert an integer to a roman numeral.
Next: Write a Python class to find validity of a string of parentheses, '(', ')', '{', '}', '[' and ']. These brackets must be close in the correct order,
for example "()" and "()[]{}" are valid but "[)", "({[)]" and "{{{" are invalid.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/class-exercises/python-class-exercise-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics