Python: Validity of a string of parentheses
Python Class: Exercise-3 with Solution
Write a Python class to check the validity of a string of parentheses, '(', ')', '{', '}', '[' and ']. These brackets must be closed in the correct order,
for example "()" and "()[]{}" are valid but "[)", "({[)]" and "{{{" are invalid.
Sample Solution:
Python Code:
class py_solution:
def is_valid_parenthese(self, str1):
stack, pchar = [], {"(": ")", "{": "}", "[": "]"}
for parenthese in str1:
if parenthese in pchar:
stack.append(parenthese)
elif len(stack) == 0 or pchar[stack.pop()] != parenthese:
return False
return len(stack) == 0
print(py_solution().is_valid_parenthese("(){}[]"))
print(py_solution().is_valid_parenthese("()[{)}"))
print(py_solution().is_valid_parenthese("()"))
Sample Output:
True False True
Pictorial Presentation:
Flowchart:
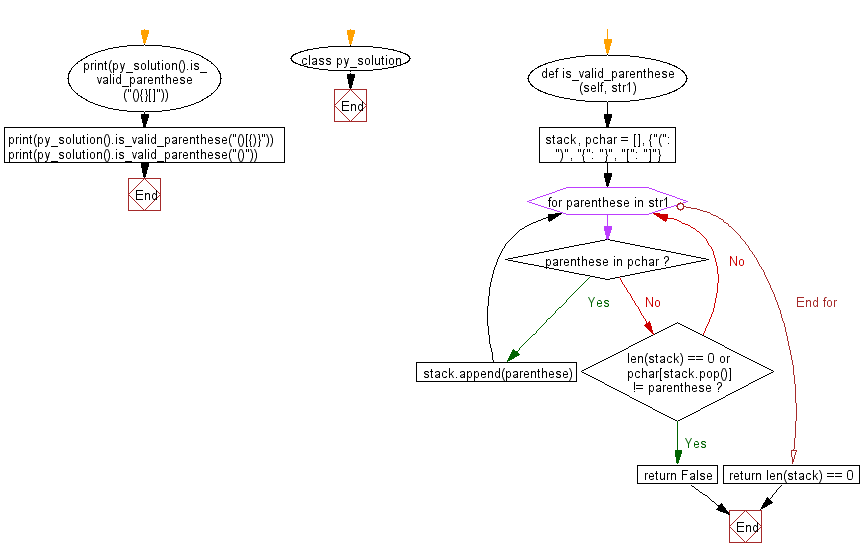
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python class to convert a roman numeral to an integer.
Next: Write a Python class to get all possible unique subsets from a set of distinct integers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/class-exercises/python-class-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics