Python: Find a pair of elements from a given array whose sum equals a specific target number
Python Class: Exercise-5 with Solution
Write a Python class to find a pair of elements (indices of the two numbers) from a given array whose sum equals a specific target number.
Difficulty: Medium. Company: Google, Facebook
Sample Solution-1:
Python Code:
class py_solution:
def twoSum(self, nums, target):
lookup = {}
for i, num in enumerate(nums):
if target - num in lookup:
return (lookup[target - num], i )
lookup[num] = i
print("index1=%d, index2=%d" % py_solution().twoSum((10,20,10,40,50,60,70),50))
Sample Output:
index1=2, index2=3
Pictorial Presentation:
Flowchart:
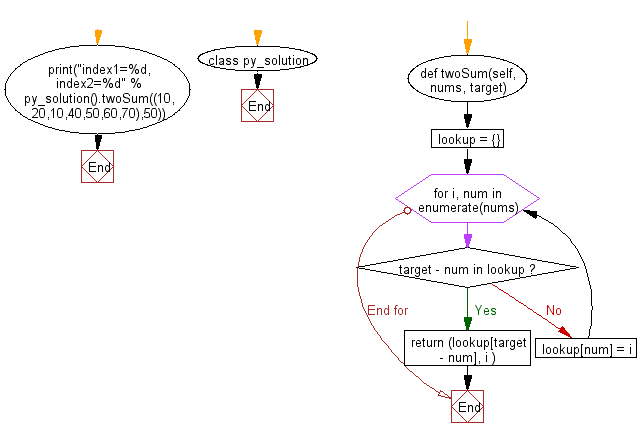
Sample Solution-2:
Python Code:
class py_solution(object):
def twoSum(self, nums, target_num):
"""
:type nums: list[int]
:type target: int
:return type: list[int]
"""
result_dict = dict()
pos = 0
while pos < len(nums):
if (target_num - nums[pos]) not in result_dict:
result_dict[nums[pos]] = pos
pos += 1
else:
return [result_dict[target_num - nums[pos]], pos]
print(py_solution().twoSum([10,20,10,40,50,60,70],50))
print(py_solution().twoSum([10,20,10,40,50,60,70],52))
Sample Output:
[2, 3] None
Flowchart:
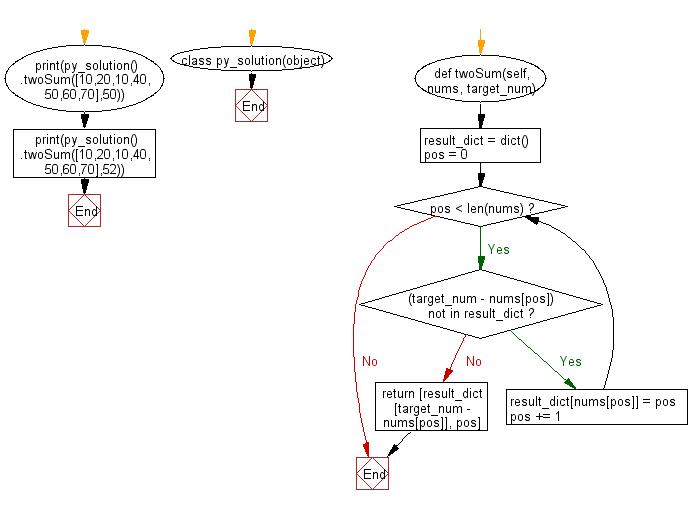
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python class to get all possible unique subsets from a set of distinct integers.
Next: Write a Python class to find the three elements that sum to zero from a set (array) of n real numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/class-exercises/python-class-exercise-5.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics