Python: Implement pow(x, n)
Python Class: Exercise-7 with Solution
Write a Python class to implement pow(x, n).
Sample Solution:
Python Code:
class py_solution:
def pow(self, x, n):
if x==0 or x==1 or n==1:
return x
if x==-1:
if n%2 ==0:
return 1
else:
return -1
if n==0:
return 1
if n<0:
return 1/self.pow(x,-n)
val = self.pow(x,n//2)
if n%2 ==0:
return val*val
return val*val*x
print(py_solution().pow(2, -3));
print(py_solution().pow(3, 5));
print(py_solution().pow(100, 0));
Sample Output:
0.125 243 1
Pictorial Presentation:
Flowchart:
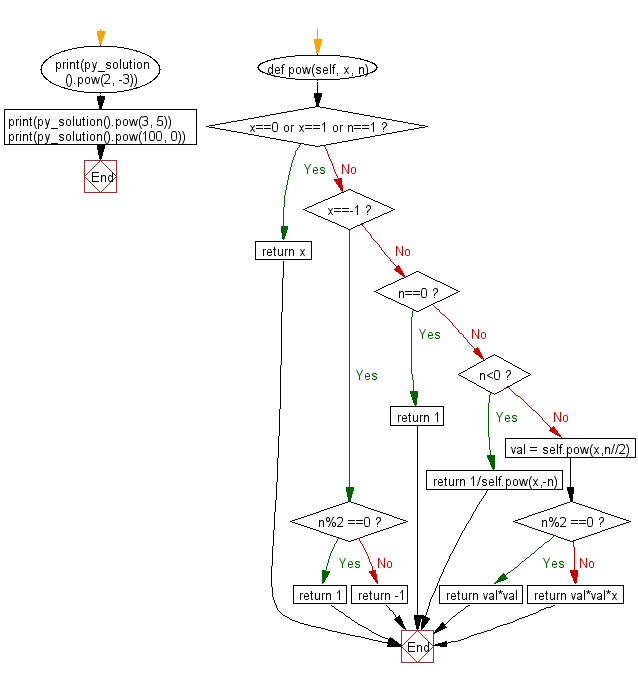
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Python class to find the three elements that sum to zero from a set (array) of n real numbers.
Next: Write a Python class to reverse a string word by word.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/class-exercises/python-class-exercise-7.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics