Python Class - Restaurant Management System
Python Class Real-life Problem: Exercise-2 with Solution
Write a Python class Restaurant with attributes like menu_items, book_table, and customer_orders, and methods like add_item_to_menu, book_tables, and customer_order.
Perform the following tasks now:
- Now add items to the menu.
- Make table reservations.
- Take customer orders.
- Print the menu.
- Print table reservations.
- Print customer orders.
Sample Solution:
Python Code:
class Restaurant:
def __init__(self):
self.menu_items = {}
self.book_table = []
self.customer_orders = []
def add_item_to_menu(self, item, price):
self.menu_items[item] = price
def book_tables(self, table_number):
self.book_table.append(table_number)
def customer_order(self, table_number, order):
order_details = {'table_number': table_number, 'order': order}
self.customer_orders.append(order_details)
def print_menu_items(self):
for item, price in self.menu_items.items():
print("{}: {}".format(item, price))
def print_table_reservations(self):
for table in self.book_table:
print("Table {}".format(table))
def print_customer_orders(self):
for order in self.customer_orders:
print("Table {}: {}".format(order['table_number'], order['order']))
restaurant = Restaurant()
# Add items
restaurant.add_item_to_menu("Cheeseburger", 9.99)
restaurant.add_item_to_menu("Caesar Salad", 8)
restaurant.add_item_to_menu("Grilled Salmon", 19.99)
restaurant.add_item_to_menu("French Fries", 3.99)
restaurant.add_item_to_menu("Fish & Chips:", 15)
# Book table
restaurant.book_tables(1)
restaurant.book_tables(2)
restaurant.book_tables(3)
# Order items
restaurant.customer_order(1, "Cheeseburger")
restaurant.customer_order(1, "Grilled Salmon")
restaurant.customer_order(2, "Fish & Chips")
restaurant.customer_order(2, "Grilled Salmon")
print("\nPopular dishes in the restaurant along with their prices:")
restaurant.print_menu_items()
print("\nTable reserved in the Restaurant:")
restaurant.print_table_reservations()
print("\nPrint customer orders:")
restaurant.print_customer_orders()
Sample Output:
Popular dishes in the restaurant along with their prices: Cheeseburger: 9.99 Caesar Salad: 8 Grilled Salmon: 19.99 French Fries: 3.99 Fish & Chips:: 15 Table reserved in the Restaurant: Table 1 Table 2 Table 3 Print customer orders: Table 1: Cheeseburger Table 1: Grilled Salmon Table 2: Fish & Chips Table 2: Grilled Salmon
Flowchart:
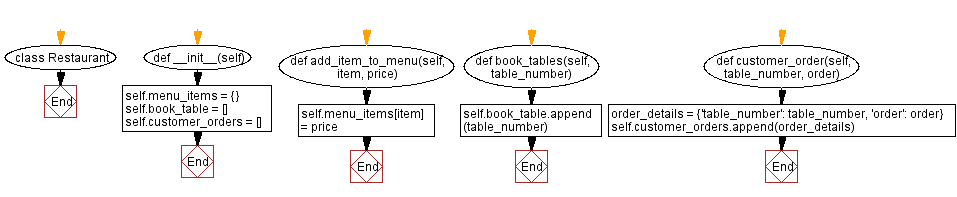
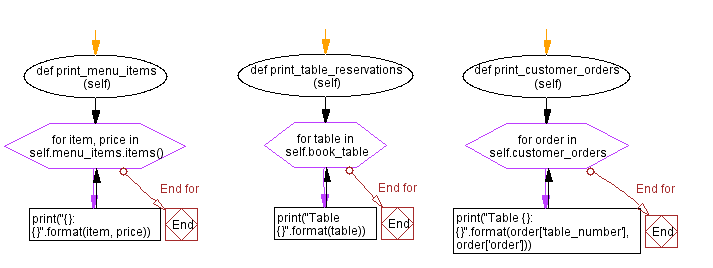
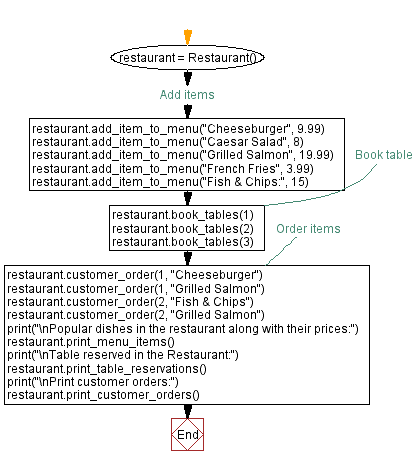
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Employee Management System.
Next: Restaurant Management System.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/class-exercises/python-class-real-life-problem-2.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics