Python Class - Bank Account Management
Python Class Real-life Problem: Exercise-3 with Solution
Write a Python class BankAccount with attributes like account_number, balance, date_of_opening and customer_name, and methods like deposit, withdraw, and check_balance.
Sample Solution:
Python Code:
class BankAccount:
def __init__(self, account_number, date_of_opening, balance, customer_name):
self.account_number = account_number
self.date_of_opening = date_of_opening
self.balance = balance
self.customer_name = customer_name
def deposit(self, amount):
self.balance += amount
print(f"${amount} has been deposited in your account.")
def withdraw(self, amount):
if amount > self.balance:
print("Insufficient balance.")
else:
self.balance -= amount
print(f"${amount} has been withdrawn from your account.")
def check_balance(self):
print(f"Current balance is ${self.balance}.")
def print_customer_details(self):
print("Name:", self.customer_name)
print("Account Number:", self.account_number)
print("Date of opening:", self.date_of_opening)
print(f"Balance: ${self.balance}\n")
# Input customer details
ac_no_1 = BankAccount(2345, "01-01-2011", 1000, "Toninho Takeo")
ac_no_2 = BankAccount(1234, "11-03-2011", 2000, "Astrid Rugile")
ac_no_3 = BankAccount(2312, "12-01-2009", 3000, "Orli Kerenza")
ac_no_4 = BankAccount(1395, "01-01-2011", 3000, "Luciana Chika")
ac_no_5 = BankAccount(6345, "01-05-2011", 4000, "Toninho Takeo")
print("Customer Details:")
ac_no_1.print_customer_details()
ac_no_2.print_customer_details()
ac_no_3.print_customer_details()
ac_no_4.print_customer_details()
ac_no_5.print_customer_details()
print("=============================")
ac_no_4.print_customer_details()
# Current balance is $3000.
# $1000 has been deposited in your account.
ac_no_4.deposit(1000)
ac_no_4.check_balance()
# Your current balance $4000.
# You want to withdraw $5000
ac_no_4.withdraw(5000)
# Output:
# Insufficient balance.
#The customer withdraw $3400.
ac_no_4.withdraw(3400)
ac_no_4.check_balance()
Sample Output:
Customer Details: Name: Toninho Takeo Account Number: 2345 Date of opening: 01-01-2011 Balance: $1000 Name: Astrid Rugile Account Number: 1234 Date of opening: 11-03-2011 Balance: $2000 Name: Orli Kerenza Account Number: 2312 Date of opening: 12-01-2009 Balance: $3000 Name: Luciana Chika Account Number: 1395 Date of opening: 01-01-2011 Balance: $3000 Name: Toninho Takeo Account Number: 6345 Date of opening: 01-05-2011 Balance: $4000 ============================= Name: Luciana Chika Account Number: 1395 Date of opening: 01-01-2011 Balance: $3000 $1000 has been deposited in your account. Current balance is $4000. Insufficient balance. $3400 has been withdrawn from your account. Current balance is $600.
Flowchart:
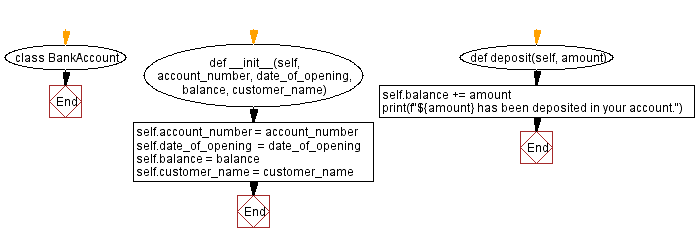
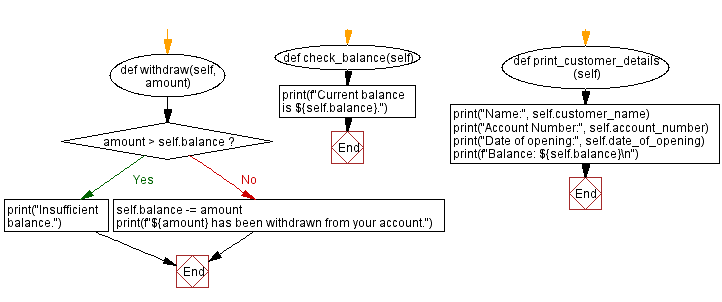
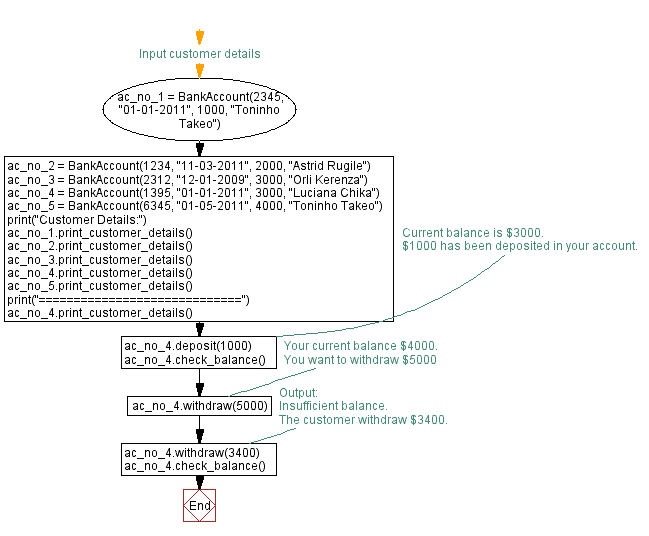
Python Code Editor:
Contribute your code and comments through Disqus.
Previous: Restaurant Management System.
Next: Inventory Management System.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/class-exercises/python-class-real-life-problem-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics