Python: Remove all the elements of a given deque object
10. Remove All Elements from a Deque
Write a Python program to remove all the elements of a given deque object.
Sample Solution:
Python Code:
# Import the collections module to use the deque data structure
import collections
# Create a tuple 'odd_nums' containing odd numbers 1, 3, 5, 7, and 9
odd_nums = (1, 3, 5, 7, 9)
# Create a deque 'odd_deque' from the 'odd_nums' tuple
odd_deque = collections.deque(odd_nums)
# Print a message to indicate the display of the original deque with odd numbers
print("Original Deque object with odd numbers:")
# Print the 'odd_deque' containing odd numbers
print(odd_deque)
# Print a message displaying the length of the 'odd_deque'
print("Deque length: %d" % (len(odd_deque)))
# Clear all elements from the 'odd_deque'
odd_deque.clear()
# Print a message to indicate the display of the 'odd_deque' after removing all numbers
print("Deque object after removing all numbers-")
# Print the empty 'odd_deque'
print(odd_deque)
# Print a message displaying the length of the empty 'odd_deque'
print("Deque length:%d" % (len(odd_deque)))
Sample Output:
Original Deque object with odd numbers: deque([1, 3, 5, 7, 9]) Deque length: 5 Deque object after removing all numbers- deque([]) Deque length:0
Flowchart:
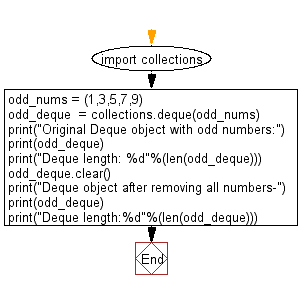
For more Practice: Solve these Related Problems:
- Write a Python program to clear a deque using the clear() method and then verify that it is empty.
- Write a Python program to remove each element from a deque one by one until it is empty, printing the deque at each step.
- Write a Python program to implement a function that empties a deque and returns the total number of removed elements.
- Write a Python program to simulate the emptying of a deque by popping from both ends until the deque is exhausted.
Go to:
Previous: Write a Python program to add more number of elements to a deque object from an iterable object.
Next: Write a Python program to copy of a deque object and verify the shallow copying process.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.