Python: Copy of a deque object and verify the shallow copying process
11. Copy a Deque and Verify Shallow Copying
Write a Python program that copies a deque object and verifies shallow copying.
Sample Solution:
Python Code:
# Import the collections module to use the deque data structure
import collections
# Create a tuple 'tup1' containing numbers 1, 3, 5, 7, and 9
tup1 = (1, 3, 5, 7, 9)
# Create a deque 'dq1' from the 'tup1' tuple
dq1 = collections.deque(tup1)
# Create a shallow copy 'dq2' of 'dq1'
dq2 = dq1.copy()
# Print a message to indicate the display of the content of 'dq1'
print("Content of dq1:")
# Print the content of 'dq1'
print(dq1)
# Print a message indicating the ID of 'dq1'
print("dq2 id:")
# Print the ID of 'dq1'
print(id(dq1))
# Print a message to indicate the display of the content of 'dq2'
print("\nContent of dq2:")
# Print the content of 'dq2'
print(dq2)
# Print a message indicating the ID of 'dq2'
print("dq2 id:")
# Print the ID of 'dq2'
print(id(dq2))
# Print a message to check if the first element of 'dq1' and 'dq2' are shallow copies
print("\nChecking the first element of dq1 and dq2 are shallow copies:")
# Print the ID of the first element of 'dq1'
print(id(dq1[0]))
# Print the ID of the first element of 'dq2'
print(id(dq2[0]))
Sample Output:
Content of dq1: deque([1, 3, 5, 7, 9]) dq2 id: 140706429557152 Content of dq2: deque([1, 3, 5, 7, 9]) dq2 id: 140706406914152 Checking the first element of dq1 and dq2 are shallow copies: 11065888 11065888
Flowchart:
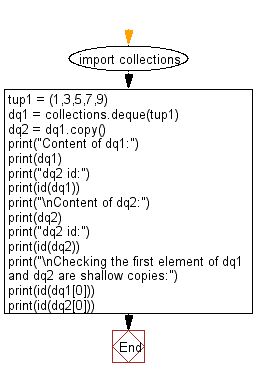
For more Practice: Solve these Related Problems:
- Write a Python program to create a shallow copy of a deque using the copy() method and compare their memory addresses.
- Write a Python program to modify a shallow copy of a deque and demonstrate that changes do not affect the original deque.
- Write a Python program to use the copy module to create a shallow copy of a deque and verify its elements are the same objects.
- Write a Python program to implement a function that returns a shallow copy of a deque and then compares the IDs of corresponding elements.
Go to:
Previous: Write a Python program to remove all the elements of a given deque object.
Next: Write a Python program to count the number of times a specific element presents in a deque object.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.