Python: Count the number of times a specific element presents in a deque object
Write a Python program to count the number of times a specific element appears in a deque object.
Sample Solution:
Python Code:
# Import the collections module to use the deque data structure
import collections
# Create a tuple 'nums' containing a sequence of numbers
nums = (2, 9, 0, 8, 2, 4, 0, 9, 2, 4, 8, 2, 0, 4, 2, 3, 4, 0)
# Create a deque 'nums_dq' from the 'nums' tuple
nums_dq = collections.deque(nums)
# Print a message to indicate the display of the count of number 2 in the sequence
print("Number of 2 in the sequence")
# Count and print the number of occurrences of 2 in 'nums_dq'
print(nums_dq.count(2))
# Print a message to indicate the display of the count of number 4 in the sequence
print("Number of 4 in the sequence")
# Count and print the number of occurrences of 4 in 'nums_dq'
print(nums_dq.count(4))
Sample Output:
Number of 2 in the sequence 5 Number of 4 in the sequence 4
Flowchart:
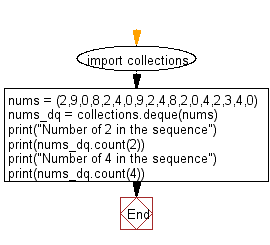
Python Code Editor:
Previous: Write a Python program to copy of a deque object and verify the shallow copying process.
Next: Write a Python program to rotate a Deque Object specified number (positive) of times.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics