Python: Rotate a Deque Object specified number (negative) of times
Python Collections: Exercise-14 with Solution
Write a Python program to rotate a deque Object a specified number (negative) of times.
Sample Solution:
Python Code:
# Import the collections module to use the deque data structure
import collections
# Declare an empty deque object named 'dq_object'
dq_object = collections.deque()
# Add elements to the deque from left to right
dq_object.append(2)
dq_object.append(4)
dq_object.append(6)
dq_object.append(8)
dq_object.append(10)
# Print a message to indicate the display of the deque before rotation
print("Deque before rotation:")
# Print the content of 'dq_object'
print(dq_object)
# Rotate the deque once in the negative direction (to the left)
dq_object.rotate(-1)
# Print a message to indicate the display of the deque after 1 negative rotation
print("\nDeque after 1 negative rotation:")
# Print the content of 'dq_object' after one negative rotation
print(dq_object)
# Rotate the deque twice in the negative direction (to the left)
dq_object.rotate(-2)
# Print a message to indicate the display of the deque after 2 negative rotations
print("\nDeque after 2 negative rotations:")
# Print the content of 'dq_object' after two negative rotations
print(dq_object)
Sample Output:
Deque before rotation: deque([2, 4, 6, 8, 10]) Deque after 1 negative rotation: deque([4, 6, 8, 10, 2]) Deque after 2 negative rotations: deque([8, 10, 2, 4, 6])
Flowchart:
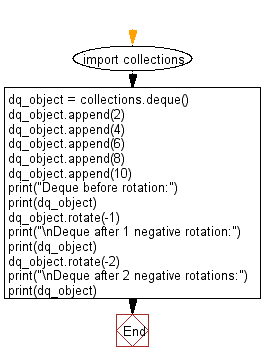
Python Code Editor:
Previous: Write a Python program to rotate a Deque Object specified number (positive) of times.
Next: Write a Python program to find the most common element of a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://198.211.115.131/python-exercises/collections/python-collections-exercise-14.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics