Python: Find the most common elements and their counts of a specified text
Write a Python program to find the most common elements and their counts in a specified text.
Sample Solution:
Python Code:
# Import the Counter class from the collections module
from collections import Counter
# Create a string 's' containing a sequence of characters
s = 'lkseropewdssafsdfafkpwe'
# Print the original string
print("Original string: " + s)
# Print a message indicating that we are going to find the most common three characters in the string
print("Most common three characters of the said string:")
# Use Counter to count the occurrences of each character in the string 's' and then find the three most common characters
print(Counter(s).most_common(3))
Sample Output:
Original string: lkseropewdssafsdfafkpwe Most common three characters of the said string: [('s', 4), ('e', 3), ('f', 3)]
Flowchart:
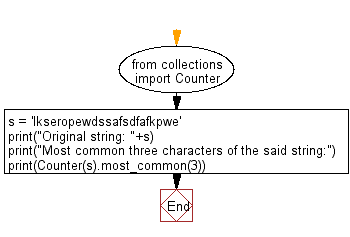
Python Code Editor:
Previous: Write a Python program that iterate over elements repeating each as many times as its count.
Next: Write a Python program to create a new deque with three items and iterate over the deque's elements.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics